esp32 用arduino连接mqtt代码
时间: 2023-11-30 12:03:53 浏览: 51
下面是一个简单的ESP32连接MQTT服务器的Arduino代码示例:
首先,你需要安装一个MQTT库。在Arduino IDE中,进入 "工具" -> "管理库",搜索 "PubSubClient" 并安装。
然后,你可以使用以下代码作为起点:
```c++
#include <WiFi.h>
#include <PubSubClient.h>
// Replace with your network credentials
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// Replace with your MQTT broker IP address
const char* mqtt_server = "your_MQTT_broker_IP";
WiFiClient espClient;
PubSubClient client(espClient);
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
client.setServer(mqtt_server, 1883);
}
void loop() {
if (!client.connected()) {
reconnect();
}
client.loop();
}
void reconnect() {
while (!client.connected()) {
Serial.println("Connecting to MQTT broker...");
if (client.connect("ESP32Client")) {
Serial.println("Connected to MQTT broker");
// Subscribe to topic
client.subscribe("test_topic");
} else {
Serial.print("Failed to connect to MQTT broker with state ");
Serial.print(client.state());
delay(2000);
}
}
}
void callback(char* topic, byte* payload, unsigned int length) {
Serial.print("Message received on topic: ");
Serial.println(topic);
Serial.print("Message content: ");
for (int i = 0; i < length; i++) {
Serial.print((char)payload[i]);
}
Serial.println();
// Add your custom logic here
}
```
该代码连接到WiFi网络,然后连接到MQTT服务器并订阅一个主题。如果连接失败,则尝试重新连接。回调函数 `callback()` 可以在收到消息时添加自定义逻辑。
你需要将以下变量替换为你自己的值:
- `ssid` 和 `password` - 你的WiFi网络名称和密码
- `mqtt_server` - 你的MQTT服务器IP地址
然后,你可以使用 `client.publish()` 函数向MQTT服务器发布消息,例如:
```c++
client.publish("test_topic", "Hello from ESP32");
```
这将发布一个 "Hello from ESP32" 的消息到 "test_topic" 主题。
相关推荐
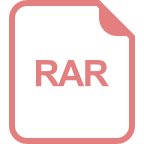














