spyder基于蚁群算法求解全球所有国家首都的TSP问题并用Cartopy库画出相应地图
时间: 2024-02-29 11:57:06 浏览: 167
为了求解全球所有国家首都的 TSP 问题,可以使用 Python 的蚁群算法库 Ant Colony Optimization (ACO)。以下是实现步骤:
1. 安装 ACO 库和相关依赖库:
```
pip install ant-colony
pip install pandas
pip install numpy
pip install cartopy
```
2. 导入所需库:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
from cartopy.io.shapereader import Reader
from cartopy.feature import ShapelyFeature
from ant_colony import AntColony
```
3. 读取国家数据:
```python
url = "https://raw.githubusercontent.com/datasets/country-codes/master/data/country-codes.csv"
countries = pd.read_csv(url)
capitals = countries[countries['Capital'].notna()]
```
4. 计算城市之间的距离矩阵:
```python
def distance(lat1, lon1, lat2, lon2):
R = 6371 # 地球平均半径,单位为公里
d_lat = np.radians(lat2 - lat1)
d_lon = np.radians(lon2 - lon1)
lat1 = np.radians(lat1)
lat2 = np.radians(lat2)
a = np.sin(d_lat / 2) ** 2 + np.cos(lat1) * np.cos(lat2) * np.sin(d_lon / 2) ** 2
c = 2 * np.arctan2(np.sqrt(a), np.sqrt(1 - a))
distance = R * c
return distance
n = len(capitals)
distances = np.zeros((n, n))
for i in range(n):
for j in range(n):
distances[i, j] = distance(capitals.iloc[i]['Latitude'], capitals.iloc[i]['Longitude'],
capitals.iloc[j]['Latitude'], capitals.iloc[j]['Longitude'])
```
5. 创建蚁群算法对象,设置参数:
```python
colony = AntColony(distances, n_ants=10, n_best=5, n_iterations=500, decay=0.1, alpha=1.0, beta=2.0)
```
6. 运行蚁群算法:
```python
shortest_path, cost = colony.run()
```
7. 将城市坐标转换为地图上的坐标,绘制地图:
```python
fig = plt.figure(figsize=(16, 12))
ax = plt.axes(projection=ccrs.PlateCarree())
ax.set_extent([-180, 180, -60, 90], crs=ccrs.PlateCarree())
ax.stock_img()
ax.add_feature(ShapelyFeature(Reader('admin_0_countries.shp').geometries(),
ccrs.PlateCarree(), facecolor='none', edgecolor='gray'))
for i in range(n):
plt.plot(capitals.iloc[i]['Longitude'], capitals.iloc[i]['Latitude'], 'bo', markersize=5, transform=ccrs.Geodetic())
for i in range(n - 1):
plt.plot([capitals.iloc[shortest_path[i]]['Longitude'], capitals.iloc[shortest_path[i+1]]['Longitude']],
[capitals.iloc[shortest_path[i]]['Latitude'], capitals.iloc[shortest_path[i+1]]['Latitude']],
'r-', linewidth=1, transform=ccrs.Geodetic())
plt.plot([capitals.iloc[shortest_path[0]]['Longitude'], capitals.iloc[shortest_path[-1]]['Longitude']],
[capitals.iloc[shortest_path[0]]['Latitude'], capitals.iloc[shortest_path[-1]]['Latitude']],
'r-', linewidth=1, transform=ccrs.Geodetic())
plt.show()
```
绘制出来的地图上,蓝色的点表示所有国家的首都,红线表示蚁群算法求解出的最短路径。
阅读全文
相关推荐
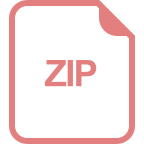













