用JSON实现省市县三级联动
时间: 2023-07-18 21:29:50 浏览: 154
可以通过以下步骤使用JSON实现省市县三级联动:
1. 准备数据
首先需要准备一份包含省市县信息的JSON数据,格式如下:
```
{
"provinceList":[
{
"id":"110000",
"name":"北京市",
"cityList":[
{
"id":"110100",
"name":"市辖区",
"countyList":[
{
"id":"110101",
"name":"东城区"
},
{
"id":"110102",
"name":"西城区"
},
...
]
},
{
"id":"110200",
"name":"县",
"countyList":[
{
"id":"110228",
"name":"密云县"
},
{
"id":"110229",
"name":"延庆县"
}
]
}
]
},
{
"id":"120000",
"name":"天津市",
"cityList":[
{
"id":"120100",
"name":"市辖区",
"countyList":[
{
"id":"120101",
"name":"和平区"
},
{
"id":"120102",
"name":"河东区"
},
...
]
},
...
]
},
...
]
}
```
2. 加载数据
使用JavaScript的XMLHttpRequest对象或jQuery的ajax方法加载JSON数据。
```
var xhr = new XMLHttpRequest();
xhr.open('GET', 'data.json', true);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
var data = JSON.parse(xhr.responseText);
// TODO: 处理数据
}
};
xhr.send();
```
3. 绑定事件
绑定省、市、县三个下拉框的change事件,当省或市的值发生变化时,重新渲染市或县的下拉框。
```
var provinceEl = document.getElementById('province');
var cityEl = document.getElementById('city');
var countyEl = document.getElementById('county');
provinceEl.addEventListener('change', function() {
var provinceId = this.value;
var province = data.provinceList.find(function(item) {
return item.id === provinceId;
});
renderCity(province.cityList);
});
cityEl.addEventListener('change', function() {
var cityId = this.value;
var city = currentProvince.cityList.find(function(item) {
return item.id === cityId;
});
renderCounty(city.countyList);
});
```
4. 渲染下拉框
根据加载的JSON数据,渲染省、市、县三个下拉框。
```
function renderProvince(provinceList) {
provinceList.forEach(function(item) {
var option = document.createElement('option');
option.value = item.id;
option.text = item.name;
provinceEl.appendChild(option);
});
}
function renderCity(cityList) {
cityEl.innerHTML = '';
countyEl.innerHTML = '';
currentProvince = {
cityList: cityList
};
cityList.forEach(function(item) {
var option = document.createElement('option');
option.value = item.id;
option.text = item.name;
cityEl.appendChild(option);
});
}
function renderCounty(countyList) {
countyEl.innerHTML = '';
countyList.forEach(function(item) {
var option = document.createElement('option');
option.value = item.id;
option.text = item.name;
countyEl.appendChild(option);
});
}
```
以上就是用JSON实现省市县三级联动的基本步骤。
阅读全文
相关推荐


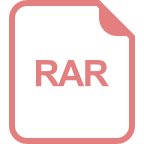
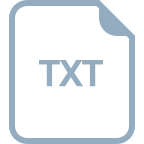
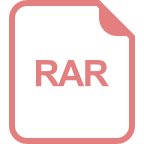
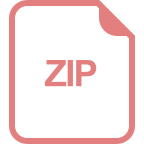
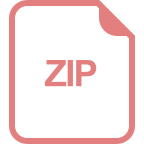


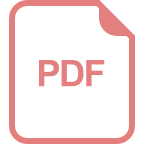
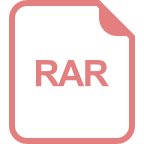

