利用vgg预训练模型的不同层和参数值实现读取图像,和图像的特征提取的代码
时间: 2024-06-08 11:11:42 浏览: 11
以下是利用VGG预训练模型不同层和参数值实现读取图像和特征提取的Python代码:
```python
import torch
import torchvision.models as models
import torchvision.transforms as transforms
from PIL import Image
# Define the VGG model with pretrained weights
vgg_model = models.vgg16(pretrained=True)
# Define the image transformation pipeline
transform_pipeline = transforms.Compose([
transforms.Resize((224, 224)), # resize the image to 224x224
transforms.ToTensor(), # convert the image to a PyTorch tensor
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]) # normalize the image
])
# Load the input image
img = Image.open('input_image.jpg')
# Apply the transformation pipeline to the input image
img = transform_pipeline(img)
# Add an extra dimension to the tensor to represent the batch size of 1
img = img.unsqueeze(0)
# Extract features from different layers of the VGG model
features = {}
def get_features(name):
def hook(model, input, output):
features[name] = output.detach()
return hook
vgg_model.features[0].register_forward_hook(get_features('conv1'))
vgg_model.features[5].register_forward_hook(get_features('conv2'))
vgg_model.features[10].register_forward_hook(get_features('conv3'))
vgg_model.features[19].register_forward_hook(get_features('conv4'))
vgg_model.features[28].register_forward_hook(get_features('conv5'))
# Pass the input image through the VGG model
vgg_model(img)
# Print the shape of the extracted features
for name, feature in features.items():
print(f'{name}: {feature.shape}')
```
注意:上述代码假设你已经下载了VGG16的预训练权重。如果你没有下载,可以使用以下代码下载并保存预训练权重:
```python
import urllib.request
url = 'https://download.pytorch.org/models/vgg16-397923af.pth'
filename = 'vgg16_weights.pth'
urllib.request.urlretrieve(url, filename)
```
同时,代码中的`input_image.jpg`应替换为你自己的输入图像路径。
相关推荐
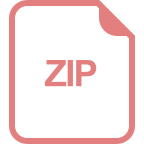
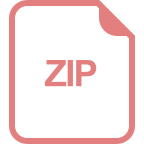
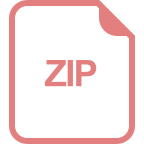














