用julia语言写一个时间序列程序
时间: 2023-12-04 16:04:29 浏览: 126
以下是一个简单的时间序列程序,使用Julia语言编写。
```julia
using TimeSeries
# 创建时间序列对象
data = TimeArray([1, 2, 3, 4, 5], Date(2021, 1, 1):Day(1):Date(2021, 1, 5))
# 打印数据
println(data)
# 计算移动平均值
ma_data = ma(data, 3)
# 打印移动平均值
println(ma_data)
```
这个程序创建了一个包含5个数据点的时间序列,从2021年1月1日开始,每天增加一个数据点,直到2021年1月5日结束。然后,程序计算了这个时间序列的3天移动平均值,并打印出了原始数据和移动平均值。
相关问题
julia语言写一个时间序列模型
以下是一个简单的时间序列模型,使用ARIMA模型进行预测:
```julia
using TimeSeries, Plots
# 生成随机时间序列
data = rand(100)
# 将数据转换为时间序列类型
ts = TimeArray(data)
# 绘制时间序列图
plot(ts)
# 拟合ARIMA模型
model = ARIMA(ts, (1,0,1))
# 预测未来10个时间步长
forecast = predict(model, 10)
# 绘制预测结果
plot(ts, label="Data")
plot!(forecast, label="Forecast")
```
在这个例子中,我们使用了TimeSeries.jl和Plots.jl库来生成一个随机时间序列并绘制时间序列图。然后,我们使用ARIMA模型对时间序列进行拟合,并使用predict函数预测未来10个时间步长。最后,我们使用plot函数绘制原始数据和预测结果。
用julia写一个时间驱动量化交易程序
以下是一个简单的时间驱动量化交易程序的Julia代码示例:
```julia
using DataFrames
using Random
# 生成随机价格数据
function generate_prices(n_days::Int)
prices = rand(100:200, n_days)
return prices
end
# 简单的交易策略:如果今天的价格比昨天高,则买入;否则卖出
function simple_strategy(prices::Vector{Float64}, position::Bool)
yesterday_price = prices[length(prices)-1]
today_price = prices[length(prices)]
if today_price > yesterday_price && !position
return true # 买入
elseif today_price < yesterday_price && position
return false # 卖出
else
return position # 保持原有仓位
end
end
# 主函数
function main(n_days::Int)
# 生成价格数据
prices = generate_prices(n_days)
# 初始化交易记录
trades = DataFrame(Date = Date[], Price = Float64[], Position = Bool[])
# 初始化初始仓位
position = false
# 循环每一天,执行交易策略并记录交易
for i in 1:n_days
position = simple_strategy(prices[1:i], position)
if position
push!(trades, (Date = Dates.today(), Price = prices[i], Position = true))
else
push!(trades, (Date = Dates.today(), Price = prices[i], Position = false))
end
end
# 打印交易记录
println(trades)
end
# 运行程序
main(10)
```
在这个简单的交易策略中,我们只是比较了今天的价格和昨天的价格,如果今天的价格比昨天高,则买入;否则卖出。实际的交易策略可能需要更加复杂的条件和算法才能达到更好的效果。此外,我们还可以添加更多的交易规则和风险管理策略来优化交易策略。
阅读全文
相关推荐
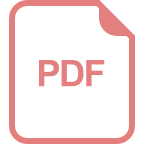
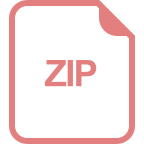
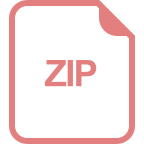













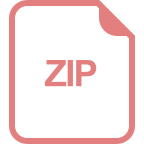