C#定时读取指定文件夹里面所有csv数据
时间: 2023-09-20 09:04:42 浏览: 167
在C#中,你可以使用`System.IO`命名空间下的类来实现定时读取指定文件夹里的所有CSV数据。以下是一个示例代码:
```csharp
using System;
using System.IO;
using System.Timers;
class Program
{
static void Main(string[] args)
{
string folderPath = @"C:\Path\To\Your\Folder"; // 指定文件夹路径
int interval = 5000; // 定时器触发间隔(毫秒)
Timer timer = new Timer(interval);
timer.Elapsed += (sender, e) => ReadCSVFiles(folderPath);
timer.Start();
Console.WriteLine("Press any key to stop the program...");
Console.ReadKey();
timer.Stop();
}
static void ReadCSVFiles(string folderPath)
{
string[] csvFiles = Directory.GetFiles(folderPath, "*.csv"); // 获取文件夹中所有CSV文件
foreach (string csvFile in csvFiles)
{
// 读取CSV文件的逻辑
Console.WriteLine("Reading file: " + csvFile);
// 使用StreamReader或CsvHelper等库读取CSV文件内容
// 处理CSV数据
// 输出处理结果
Console.WriteLine("Finished reading file: " + csvFile);
}
}
}
```
上述代码使用了`System.Timers.Timer`类来实现定时触发读取操作。你可以根据自己的需求修改`folderPath`和`interval`变量的值,并在`ReadCSVFiles`方法中实现对CSV文件的读取和处理逻辑。
阅读全文
相关推荐
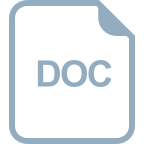
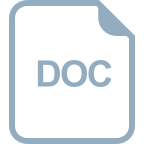
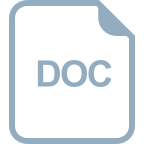
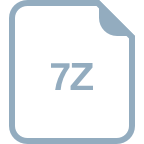
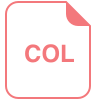
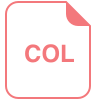

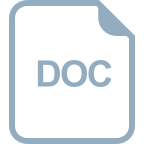
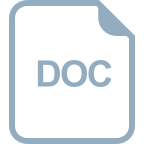
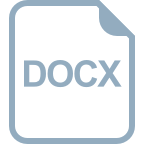
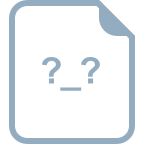
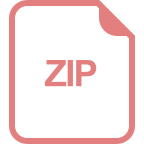
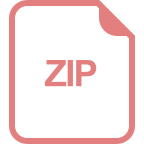