AttributeError: 'numpy.ndarray' object has no attribute 'extend'
时间: 2023-09-13 07:05:50 浏览: 97
This error occurs when you try to use the `extend()` method on a NumPy array. NumPy arrays do not have an `extend()` method, as they are not designed to be extended dynamically like Python lists.
To fix this error, you can either convert the NumPy array to a Python list and then use the `extend()` method, or use NumPy methods like `concatenate()` or `append()` to add elements to the array.
Here's an example of how to convert a NumPy array to a Python list and use the `extend()` method:
```
import numpy as np
# Create a NumPy array
arr = np.array([1, 2, 3])
# Convert the array to a list
lst = arr.tolist()
# Use the extend() method on the list
lst.extend([4, 5, 6])
# Convert the list back to a NumPy array
arr = np.array(lst)
```
Alternatively, you can use NumPy methods to add elements to the array:
```
import numpy as np
# Create a NumPy array
arr = np.array([1, 2, 3])
# Use concatenate() to add elements to the array
arr = np.concatenate((arr, [4, 5, 6]))
# Use append() to add a single element to the array
arr = np.append(arr, 7)
```
阅读全文
相关推荐
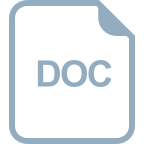
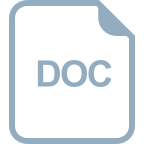
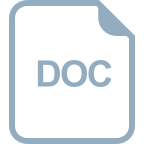





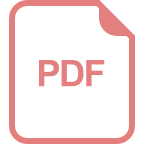







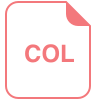

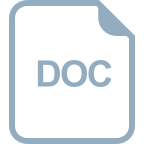