生成一个生产者消费者问题c语言代码
时间: 2023-09-28 17:07:46 浏览: 155
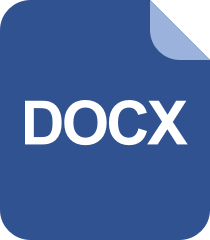
利用数组解决生产者消费者问题
好的,以下是生成一个生产者消费者问题c语言代码的示例:
```
#include <stdio.h>
#include <pthread.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int in = 0;
int out = 0;
int count = 0;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t full = PTHREAD_COND_INITIALIZER;
pthread_cond_t empty = PTHREAD_COND_INITIALIZER;
void *producer(void *arg) {
int i;
for (i = 0; i < 20; i++) {
pthread_mutex_lock(&mutex);
while (count == BUFFER_SIZE) {
pthread_cond_wait(&empty, &mutex);
}
buffer[in] = i;
in = (in + 1) % BUFFER_SIZE;
count++;
pthread_cond_signal(&full);
pthread_mutex_unlock(&mutex);
printf("Produced: %d\n", i);
}
}
void *consumer(void *arg) {
int i;
for (i = 0; i < 20; i++) {
pthread_mutex_lock(&mutex);
while (count == 0) {
pthread_cond_wait(&full, &mutex);
}
int item = buffer[out];
out = (out + 1) % BUFFER_SIZE;
count--;
pthread_cond_signal(&empty);
pthread_mutex_unlock(&mutex);
printf("Consumed: %d\n", item);
}
}
int main() {
pthread_t prod, cons;
pthread_create(&prod, NULL, producer, NULL);
pthread_create(&cons, NULL, consumer, NULL);
pthread_join(prod, NULL);
pthread_join(cons, NULL);
return 0;
}
```
这段代码实现了一个简单的生产者-消费者问题。它使用了一个循环缓冲区,两个线程分别充当生产者和消费者。它通过互斥锁和条件变量来保证缓冲区被正确地访问和同步。
阅读全文
相关推荐
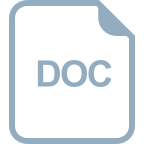
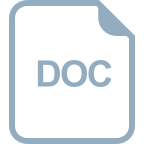
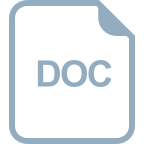














