java用ArrayList存储商品并找出集合中价格最高的商品代码
时间: 2023-08-31 21:23:13 浏览: 179
### 回答1:
可以使用以下代码找出集合中价格最高的商品:
ArrayList<Product> products = new ArrayList<Product>();
double maxPrice = 0;
Product mostExpensiveProduct = null;
for(Product product : products){
if(product.getPrice() > maxPrice){
maxPrice = product.getPrice();
mostExpensiveProduct = product;
}
}
### 回答2:
Java中可以使用ArrayList来存储商品对象,并通过遍历集合中的商品对象的价格属性,来找出价格最高的商品代码。
首先,我们需要创建一个商品类,该类包含商品代码和价格属性。代码样例如下:
```java
public class Product {
private String code;
private double price;
public Product(String code, double price) {
this.code = code;
this.price = price;
}
public String getCode() {
return code;
}
public double getPrice() {
return price;
}
}
```
接下来,我们可以创建一个ArrayList,并将商品对象添加到集合中,代码样例如下:
```java
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<Product> products = new ArrayList<>();
// 将商品对象添加到集合中
products.add(new Product("P001", 10.99));
products.add(new Product("P002", 19.99));
products.add(new Product("P003", 15.99));
products.add(new Product("P004", 25.99));
// 遍历集合并找出价格最高的商品代码
double maxPrice = 0;
String maxPriceProductCode = "";
for (Product p : products) {
if (p.getPrice() > maxPrice) {
maxPrice = p.getPrice();
maxPriceProductCode = p.getCode();
}
}
System.out.println("价格最高的商品代码:" + maxPriceProductCode);
}
}
```
运行以上代码,输出结果为:
```
价格最高的商品代码:P004
```
通过以上步骤,我们成功通过ArrayList存储商品,并找出集合中价格最高的商品代码。
### 回答3:
可以通过以下步骤使用ArrayList存储商品并找出集合中价格最高的商品代码。
首先,我们需要定义一个商品类,包含商品代码和价格这两个属性。代码示例如下:
```
public class Product {
private String code;
private double price;
// 构造方法
public Product(String code, double price) {
this.code = code;
this.price = price;
}
// Getter和Setter方法省略
// 重写toString方法,用于输出商品信息
@Override
public String toString() {
return "商品代码:" + code + ",价格:" + price;
}
}
```
然后,我们可以创建一个ArrayList来存储商品对象。代码示例如下:
```
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<Product> products = new ArrayList<>();
// 添加商品对象到ArrayList中
products.add(new Product("001", 9.99));
products.add(new Product("002", 19.99));
products.add(new Product("003", 29.99));
// 找出价格最高的商品代码
double maxPrice = 0;
String maxPriceCode = "";
for (Product product : products) {
if (product.getPrice() > maxPrice) {
maxPrice = product.getPrice();
maxPriceCode = product.getCode();
}
}
System.out.println("价格最高的商品代码:" + maxPriceCode);
}
}
```
在上述代码中,我们创建了一个products的ArrayList,用于存储商品对象。然后,通过遍历ArrayList找出价格最高的商品代码。最后,输出找到的最高价格的商品代码。
阅读全文
相关推荐
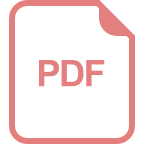
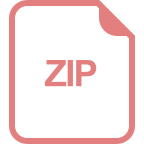
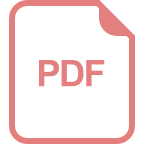
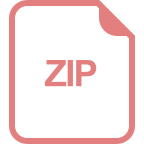
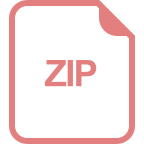
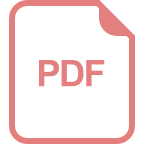
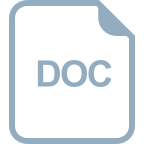
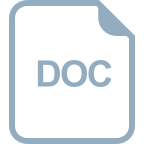
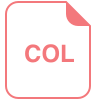
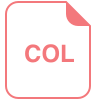








