int SeqSearch2(int r[ ], int n, int k) { 点击在此输入代码 i=n; while (r[i]!=k) 点击在此输入代码 return i; }
时间: 2024-05-17 18:17:12 浏览: 31
下面是完整的代码:
```c++
int SeqSearch2(int r[], int n, int k) {
int i = n;
r[0] = k; // 将关键字 k 存放在 r[0] 中,作为哨兵
while (r[i] != k) {
i--;
}
return i; // 返回下标,若查找失败则返回 0
}
```
在这个算法中,我们将要查找的关键字 k 存放在 r[0] 中,作为哨兵。这样,在查找时就不需要每次都判断是否已经到达了数组的边界。当查找到要查找的元素或者查找结束时(即 i=0),返回其下标即可。如果最终 i=0,说明查找失败,返回 0。
需要注意的是,在该算法中,我们修改了数组的第一个元素。因此,在实际使用时,需要确保该数组不会被其他代码使用,或者在使用完该算法后将数组恢复原状。
相关问题
以下是 哨兵排序 检查是否有误int SeqSearch2(int r[ ], int n, int k) { r[0] = k; i=n; while (r[i]!=k) --i; return i; }
你给出的代码实现中存在错误。
在这个算法中,我们将要查找的关键字 k 存放在 r[0] 中,作为哨兵。这样,在查找时就不需要每次都判断是否已经到达了数组的边界。当查找到要查找的元素或者查找结束时,返回其下标即可。如果最终 i=0,说明查找失败,返回 -1。
下面是正确的代码实现:
```c++
int SeqSearch2(int r[], int n, int k) {
r[0] = k; // 将关键字 k 存放在 r[0] 中,作为哨兵
int i = n;
while (r[i] != k) {
i--;
}
if (i == 0) {
return -1; // 查找失败,返回 -1
} else {
return i; // 返回下标
}
}
```
需要注意的是,在该算法中,我们修改了数组的第一个元素。因此,在实际使用时,需要确保该数组不会被其他代码使用,或者在使用完该算法后将数组恢复原状。
int SeqSearch(SeqList R,int n,KeyType k){ int i = 0; while (i<n && R[i].key!=k) i++; if(i>=n) return -1; else return i+1; }这段算法中,i>=n有什么作用
在该算法中,i>=n是用来判断是否查找到了整个序列R中的所有元素。当查找过程中,i<n并且R[i].key!=k时,表示还有元素需要继续查找。当i>=n时,说明已经遍历完整个序列,但是仍然没有找到关键字为k的元素,此时算法终止。因此,当函数返回值为-1时,表示查找失败,即序列中不存在关键字为k的元素。
需要注意的是,当i>=n时,i的值已经超出了序列R的下标范围,因此在返回结果时需要将其加1,从而得到元素在序列中的位置。
相关推荐
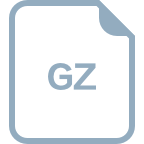
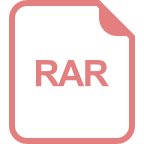













