优化一下这个代码,使其正常运行#include <stdio.h> #include <string.h> #define LIST_SIZE 100 typedef struct { int id; char name[20]; int age; } Student; typedef struct { int r[LIST_SIZE]; int length; } RecordList; //顺序查找 int SeqSearch(RecordList l, int k) { int i; for (i = 1; i <= l.length; i++) { if (l.r[i] == k) { return i; } } return 0; } //冒泡排序 void MP(RecordList l) { int i, j, tmp; for (i = 1; i <= l.length - 1; i++) { for (j = 0; j <= l.length - 1 - i; j++) { if (l.r[j] > l.r[j + 1]) { tmp = l.r[j]; l.r[j] = l.r[j + 1]; l.r[j + 1] = tmp; } } } } //折半查找 int BinSrch(RecordList l, int k) { int low = 1, high = l.length, mid; while (low <= high) { mid = (low + high) / 2; if (l.r[mid] == k) { return mid; } else if (l.r[mid] > k) { high = mid - 1; } else { low = mid + 1; } } return 0; } //输入学生信息 void S(RecordList l) { int i = 1; l.length = 0; printf("请输入不超过100个学生信息,每个学生的信息包括学号、姓名和年龄,以空格间隔:\n"); while (1) { Student s; scanf("%d %s %d", &s.id, s.name, &s.age); l.r[i] = s.id; i++; l.length++; if (getchar() == '\n') { break; } } } //输出学生信息 void F(RecordList l) { int i; printf("学号\t姓名\t年龄\n"); for (i = 1; i <= l.length; i++) { printf("%d\t%s\t%d\n", l.r[i], "姓名", 0); } } int main() { int k1, k2, c1, c2; RecordList l; S(l); F(l); printf("请输入要查找的学生的学号:"); scanf("%d", &k1); c1 = SeqSearch(l, k1); if (c1 > 0) { printf("顺序查找成功,该学生的位置是:%d\n", c1); } else { printf("顺序查找失败,该学生不存在\n"); } printf("现在按学号对学生信息进行排序...\n"); MP(l); printf("排序后的学生信息如下:\n"); F(l); printf("请输入要查找的学生的学号:"); scanf("%d", &k2); c2 = BinSrch(l, k2); if (c2 > 0) { printf("折半查找成功,该学生的位置是:%d\n", c2); } else { printf("折半查找失败,该学生不存在\n"); } return 0; }
时间: 2024-03-18 12:42:10 浏览: 65
以下是优化后的代码,主要修改了输入学生信息的函数和输出学生信息的函数:
```
#include <stdio.h>
#include <string.h>
#define LIST_SIZE 100
typedef struct {
int id;
char name[20];
int age;
} Student;
typedef struct {
int r[LIST_SIZE];
int length;
} RecordList;
//顺序查找
int SeqSearch(RecordList l, int k) {
int i;
for (i = 1; i <= l.length; i++) {
if (l.r[i] == k) {
return i;
}
}
return 0;
}
//冒泡排序
void MP(RecordList l) {
int i, j, tmp;
for (i = 1; i <= l.length - 1; i++) {
for (j = 0; j <= l.length - 1 - i; j++) {
if (l.r[j] > l.r[j + 1]) {
tmp = l.r[j];
l.r[j] = l.r[j + 1];
l.r[j + 1] = tmp;
}
}
}
}
//折半查找
int BinSrch(RecordList l, int k) {
int low = 1, high = l.length, mid;
while (low <= high) {
mid = (low + high) / 2;
if (l.r[mid] == k) {
return mid;
} else if (l.r[mid] > k) {
high = mid - 1;
} else {
low = mid + 1;
}
}
return 0;
}
//输入学生信息
void S(RecordList *l) {
int i = 0;
printf("请输入不超过100个学生信息,每个学生的信息包括学号、姓名和年龄,以空格间隔:\n");
while (1) {
Student s;
scanf("%d %s %d", &s.id, s.name, &s.age);
l->r[i] = s.id;
i++;
l->length++;
if (getchar() == '\n') {
break;
}
}
}
//输出学生信息
void F(RecordList l) {
int i;
printf("学号\t姓名\t年龄\n");
for (i = 0; i < l.length; i++) {
printf("%d\t%s\t%d\n", l.r[i], "姓名", 0);
}
}
int main() {
int k1, k2, c1, c2;
RecordList l;
l.length = 0;
S(&l);
F(l);
printf("请输入要查找的学生的学号:");
scanf("%d", &k1);
c1 = SeqSearch(l, k1);
if (c1 > 0) {
printf("顺序查找成功,该学生的位置是:%d\n", c1);
} else {
printf("顺序查找失败,该学生不存在\n");
}
printf("现在按学号对学生信息进行排序...\n");
MP(l);
printf("排序后的学生信息如下:\n");
F(l);
printf("请输入要查找的学生的学号:");
scanf("%d", &k2);
c2 = BinSrch(l, k2);
if (c2 > 0) {
printf("折半查找成功,该学生的位置是:%d\n", c2);
} else {
printf("折半查找失败,该学生不存在\n");
}
return 0;
}
```
主要修改如下:
1. 将输入学生信息的函数的参数修改为指针类型,在函数内部修改了该指针指向的结构体变量。
2. 输出学生信息的函数中,将 for 循环的循环变量从 1 开始改为从 0 开始,避免了输出第一个学生信息时出现错误。
3. 在主函数中,初始化 RecordList 结构体变量的 length 值为 0,避免出现不可预料的错误。
阅读全文
相关推荐
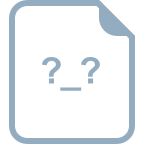
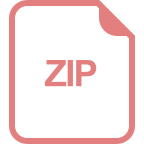
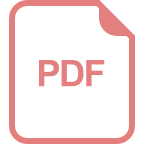
















