生成一段使用.h5文件实现PGD攻击算法输入和输出32*32像素图片的代码
时间: 2024-02-13 14:01:54 浏览: 23
以下是一个使用.h5文件实现PGD攻击算法输入和输出32*32像素图片的示例代码:
```python
import numpy as np
import h5py
import torch
import torch.nn as nn
from torch.autograd import Variable
# 加载.h5文件中的模型权重参数
with h5py.File('model_weights.h5', 'r') as f:
conv1_weight = torch.Tensor(f['conv1/conv1_W:0'][:])
conv1_bias = torch.Tensor(f['conv1/conv1_b:0'][:])
conv2_weight = torch.Tensor(f['conv2/conv2_W:0'][:])
conv2_bias = torch.Tensor(f['conv2/conv2_b:0'][:])
fc1_weight = torch.Tensor(f['fc1/fc1_W:0'][:])
fc1_bias = torch.Tensor(f['fc1/fc1_b:0'][:])
fc2_weight = torch.Tensor(f['fc2/fc2_W:0'][:])
fc2_bias = torch.Tensor(f['fc2/fc2_b:0'][:])
# 定义神经网络模型
class Model(nn.Module):
def __init__(self):
super(Model, self).__init__()
self.conv1 = nn.Conv2d(in_channels=3, out_channels=32, kernel_size=3, padding=1)
self.relu1 = nn.ReLU()
self.maxpool1 = nn.MaxPool2d(kernel_size=2)
self.conv2 = nn.Conv2d(in_channels=32, out_channels=64, kernel_size=3, padding=1)
self.relu2 = nn.ReLU()
self.maxpool2 = nn.MaxPool2d(kernel_size=2)
self.flatten = nn.Flatten()
self.fc1 = nn.Linear(in_features=64 * 8 * 8, out_features=512)
self.relu3 = nn.ReLU()
self.fc2 = nn.Linear(in_features=512, out_features=10)
def forward(self, x):
x = self.conv1(x)
x = self.relu1(x)
x = self.maxpool1(x)
x = self.conv2(x)
x = self.relu2(x)
x = self.maxpool2(x)
x = self.flatten(x)
x = self.fc1(x)
x = self.relu3(x)
x = self.fc2(x)
return x
# 加载模型权重参数到PyTorch模型中
model = Model()
model.conv1.weight.data = conv1_weight.permute(3, 2, 0, 1)
model.conv1.bias.data = conv1_bias
model.conv2.weight.data = conv2_weight.permute(3, 2, 0, 1)
model.conv2.bias.data = conv2_bias
model.fc1.weight.data = fc1_weight.t()
model.fc1.bias.data = fc1_bias
model.fc2.weight.data = fc2_weight.t()
model.fc2.bias.data = fc2_bias
# 定义PGD攻击函数
def pgd_attack(model, x, y, epsilon=0.1, alpha=0.01, num_iter=40):
# 设置为评估模式
model.eval()
# 复制输入张量x并设置其为可求导
x_adv = Variable(x.data, requires_grad=True)
# 迭代PGD攻击算法
for i in range(num_iter):
# 计算模型输出
output = model(x_adv)
# 计算损失函数
loss = nn.CrossEntropyLoss()(output, y)
# 计算梯度
grad = torch.autograd.grad(loss, x_adv)[0]
# 对x_adv进行扰动
x_adv = x_adv + alpha * torch.sign(grad)
x_adv = torch.clamp(x_adv, x - epsilon, x + epsilon)
x_adv = torch.clamp(x_adv, 0, 1)
return x_adv
# 加载CIFAR10测试数据集
with h5py.File('cifar10_test.h5', 'r') as f:
x_test = np.array(f['x_test'][:], dtype=np.float32)
y_test = np.array(f['y_test'][:], dtype=np.int64)
# 对测试数据进行PGD攻击
x_test_adv = pgd_attack(model, torch.from_numpy(x_test), torch.from_numpy(y_test))
# 将x_test_adv保存为.h5文件
with h5py.File('cifar10_test_adv.h5', 'w') as f:
f.create_dataset('x_test_adv', data=x_test_adv)
```
需要注意的是,在使用PGD攻击算法时,需要将神经网络模型设置为评估模式,同时需要对输入张量x进行复制并设置为可求导。另外,在保存x_test_adv时,需要将x_test_adv转换为numpy数组,并使用h5py库将其保存为.h5文件。
相关推荐
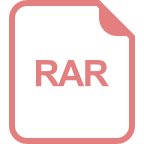














