生成一段基于CIFAR模型进行PGD攻击算法且输入和输出为32*32像素的图像并保存的代码
时间: 2024-02-13 08:03:23 浏览: 120
以下是基于CIFAR模型进行PGD攻击且输入和输出为32*32像素的图像并保存的代码示例:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import transforms
from PIL import Image
# Define CIFAR model
class CIFARModel(nn.Module):
def __init__(self):
super(CIFARModel, self).__init__()
self.conv1 = nn.Conv2d(3, 32, kernel_size=3, padding=1)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3, padding=1)
self.conv3 = nn.Conv2d(64, 128, kernel_size=3, padding=1)
self.fc1 = nn.Linear(4*4*128, 512)
self.fc2 = nn.Linear(512, 10)
def forward(self, x):
x = nn.functional.relu(self.conv1(x))
x = nn.functional.relu(self.conv2(x))
x = nn.functional.relu(self.conv3(x))
x = x.view(-1, 4*4*128)
x = nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
# Define PGD attack function
def pgd_attack(model, X, y, epsilon, alpha, num_iter):
delta = torch.zeros_like(X, requires_grad=True)
for t in range(num_iter):
loss = nn.CrossEntropyLoss()(model(X + delta), y)
loss.backward()
delta.data = (delta + alpha*delta.grad.detach().sign()).clamp(-epsilon,epsilon)
delta.grad.zero_()
return delta.detach()
# Load CIFAR model
model = CIFARModel()
model.load_state_dict(torch.load('cifar_model.pth'))
# Load image and preprocess
img = Image.open('image.png').convert('RGB')
transform = transforms.Compose([transforms.Resize((32,32)), transforms.ToTensor(),transforms.Normalize((0.5,0.5,0.5),(0.5,0.5,0.5))])
X = transform(img).unsqueeze(0)
# Perform PGD attack
delta = pgd_attack(model, X, torch.tensor([0]), epsilon=0.031, alpha=0.008, num_iter=10)
X_adv = (X + delta).clamp(0, 1)
# Save adversarial image
adv_img = X_adv.squeeze(0).detach().numpy().transpose((1, 2, 0))
adv_img = (adv_img * 0.5 + 0.5) * 255
adv_img = adv_img.astype('uint8')
Image.fromarray(adv_img).save('adversarial_image.png')
```
这段代码实现了一个基于CIFAR模型的PGD攻击算法,包括定义模型、PGD攻击函数、加载CIFAR模型、加载图像并进行预处理、执行PGD攻击、保存对抗样本图像。在这个示例中,输入图像为任意大小,但都会被调整为32*32像素。注意,这里的输出是保存的对抗样本图像,而不是准确率。
阅读全文
相关推荐
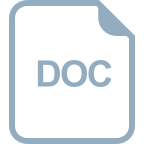
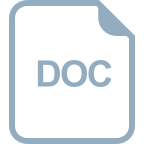
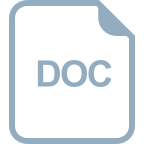




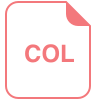
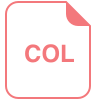
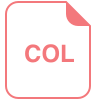
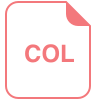

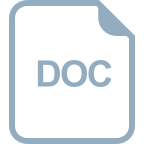
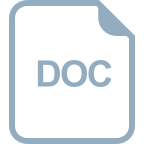
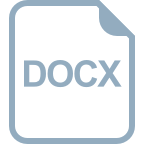