DnCnn代码讲解详细步骤‘
时间: 2023-11-12 13:58:16 浏览: 36
DnCNN(Denoising Convolutional Neural Network)是一种用于图像去噪的深度学习模型,它采用了卷积神经网络结构,可以自动学习图像中的噪声分布,从而将噪声去除。
下面是DnCNN的代码讲解详细步骤:
1.导入必要的库和模块
``` python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
import matplotlib.pyplot as plt
from torchvision import transforms, datasets
```
2.定义DnCNN模型
``` python
class DnCNN(nn.Module):
def __init__(self):
super(DnCNN, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, padding=1, bias=False)
self.bn1 = nn.BatchNorm2d(64)
self.relu = nn.ReLU(inplace=True)
self.conv_layers = nn.Sequential(*[
nn.Conv2d(64, 64, kernel_size=3, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 64, kernel_size=3, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 64, kernel_size=3, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 64, kernel_size=3, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 3, kernel_size=3, padding=1, bias=False)
])
def forward(self, x):
out = self.conv1(x)
out = self.bn1(out)
out = self.relu(out)
out = self.conv_layers(out)
return out
```
DnCNN模型的输入是带有噪声的图像,输出是去噪后的图像。
3.定义损失函数和优化器
``` python
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
```
DnCNN模型的损失函数采用均方误差(MSE)损失函数,优化器采用Adam优化器。
4.训练DnCNN模型
``` python
for epoch in range(num_epochs):
for i, (images, _) in enumerate(train_loader):
images = images.cuda()
optimizer.zero_grad()
outputs = model(images)
loss = criterion(outputs, images)
loss.backward()
optimizer.step()
if (i+1) % 10 == 0:
print ('Epoch [{}/{}], Step [{}/{}], Loss: {:.4f}'
.format(epoch+1, num_epochs, i+1, total_step, loss.item()))
```
训练DnCNN模型的过程中,首先将输入数据送入模型进行前向传播,计算模型输出和真实标签之间的损失,然后进行反向传播,更新模型参数。
5.测试DnCNN模型
``` python
model.eval()
with torch.no_grad():
for images, _ in test_loader:
images = images.cuda()
outputs = model(images)
outputs = outputs.cpu()
outputs = outputs.detach().numpy()
outputs = np.transpose(outputs, (0, 2, 3, 1))
for j in range(batch_size):
img = outputs[j,:,:,:]
img = np.clip(img, 0, 1)
plt.imshow(img)
plt.show()
```
在测试DnCNN模型时,将模型设置为评估模式(eval),不进行梯度计算,直接将测试集送入模型进行前向传播,得到模型输出,将输出转化为numpy数组,并进行后处理,最终输出去噪后的图像。
相关推荐
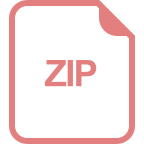
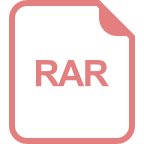














