C语言用两个队列实现栈并写出main函数
时间: 2024-12-18 22:30:17 浏览: 13
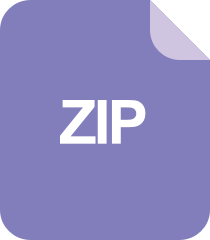
C语言课程作业栈队列实现停车场.zip
在C语言中,可以使用两个队列分别作为入栈和出栈操作的数据结构来实现一个栈的功能。这种做法通常称为“双端队列模拟栈”(Deque as Stack)。以下是简单的实现步骤和一个示例`main()`函数:
首先,我们需要包含必要的头文件,如`stdio.h`, `stdlib.h`, 和 `queue.h`(`#include <stdio.h>`, `#include <stdlib.h>`, `#include <queue.h>`)。
然后定义一个结构体用于数据存储,假设我们有一个整数类型的元素:
```c
#include <stdio.h>
#include <stdlib.h>
#include <queue.h>
typedef struct {
int value;
} StackElement;
```
接着,我们可以创建一个双向队列(`deque`)来作为我们的栈:
```c
// 创建空队列
Queue* create_stack() {
Queue *stack = (Queue*)malloc(sizeof(Queue));
stack->front = stack->rear = -1;
return stack;
}
// 入栈操作
void push(StackElement item, Queue* q) {
if (!q->rear == -1) {
Enqueue(q, (char*)&item);
} else {
printf("Stack is full.\n");
}
}
// 出栈操作
StackElement pop(Queue* q) {
if (q->front != -1) {
StackElement item;
Dequeue(q, (char*)&item);
return item;
} else {
printf("Stack is empty.\n");
return (StackElement){0}; // 返回一个默认值或者抛异常
}
}
```
最后,我们编写`main()`函数来演示栈的操作:
```c
int main() {
Queue* stack = create_stack();
// 入栈
push({1}, stack);
push({2}, stack);
push({3}, stack);
阅读全文
相关推荐
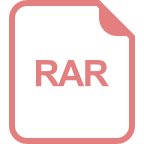
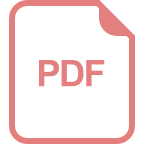







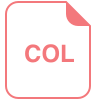
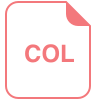






