c语言编程用消息队列、sys/msg.h里的函数,实现两个进程间的互相读写
时间: 2023-06-15 10:06:47 浏览: 100
好的,下面是一个简单的示例代码,其中包括了两个进程间的消息队列通信。其中一个进程发送消息,另一个进程接收消息。
发送进程代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct {
long type;
char text[MSG_SIZE];
} message_t;
int main() {
int msg_id;
message_t message;
char text[MSG_SIZE];
// 创建消息队列
msg_id = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if(msg_id == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
// 发送消息
printf("Enter a message: ");
fgets(text, MSG_SIZE, stdin);
message.type = 1;
strncpy(message.text, text, MSG_SIZE);
if(msgsnd(msg_id, &message, sizeof(message_t) - sizeof(long), 0) == -1) {
perror("msgsnd failed");
exit(EXIT_FAILURE);
}
printf("Message sent\n");
// 删除消息队列
if(msgctl(msg_id, IPC_RMID, NULL) == -1) {
perror("msgctl failed");
exit(EXIT_FAILURE);
}
return 0;
}
```
接收进程代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct {
long type;
char text[MSG_SIZE];
} message_t;
int main() {
int msg_id;
message_t message;
// 获取消息队列
msg_id = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if(msg_id == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
// 接收消息
if(msgrcv(msg_id, &message, sizeof(message_t) - sizeof(long), 1, 0) == -1) {
perror("msgrcv failed");
exit(EXIT_FAILURE);
}
printf("Received message: %s", message.text);
// 删除消息队列
if(msgctl(msg_id, IPC_RMID, NULL) == -1) {
perror("msgctl failed");
exit(EXIT_FAILURE);
}
return 0;
}
```
可以在两个不同的命令行窗口中运行这两个程序,分别作为发送进程和接收进程。运行后,发送进程会提示输入要发送的消息,接收进程会等待接收消息,并在接收到消息后打印出来。可以通过修改代码中的消息类型来让不同的进程之间传递不同类型的消息。
阅读全文
相关推荐
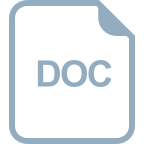
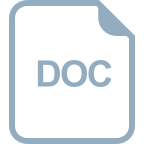


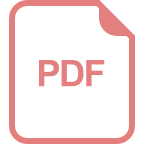
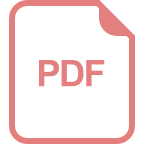
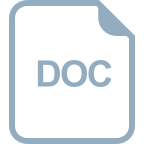
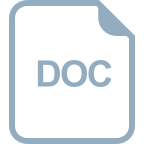
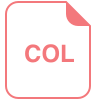
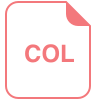


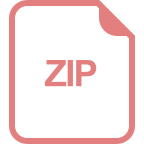
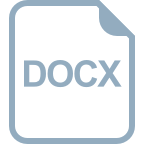