编写一个程序 message.c,在其中由父进程建立一个信息队列,然后由新建立 的子进程在表明身份后,向消息队列中写如下列信息:Hi,this is child process sending message!。父进程在等待子进程对消息队列写操作完成后,从中读取信息,并显示出来,。 在删除消息队列后结束程序,C语言代码实现加注释
时间: 2024-02-09 07:13:31 浏览: 21
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define MSG_SIZE 1024 // 定义消息的最大长度
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
int msgid;
pid_t pid;
key_t key;
// 生成key值
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(1);
}
// 创建消息队列
if ((msgid = msgget(key, IPC_CREAT|0666)) == -1) {
perror("msgget");
exit(1);
}
// 创建子进程
if ((pid = fork()) == -1) {
perror("fork");
exit(1);
}
if (pid == 0) { // 子进程
struct msgbuf buf;
buf.mtype = 1;
printf("Child process is sending message...\n");
strcpy(buf.mtext, "Hi, this is child process sending message!");
// 发送消息到消息队列
if (msgsnd(msgid, &buf, strlen(buf.mtext)+1, 0) == -1) {
perror("msgsnd");
exit(1);
}
exit(0);
} else { // 父进程
struct msgbuf buf;
// 等待子进程写入消息到消息队列
if (waitpid(pid, NULL, 0) == -1) {
perror("waitpid");
exit(1);
}
// 从消息队列中读取消息
if (msgrcv(msgid, &buf, MSG_SIZE, 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Received message: %s\n", buf.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
}
return 0;
}
```
以上代码中,通过ftok函数生成key值,创建消息队列时使用IPC_CREAT和0666参数表示如果不存在则创建,权限设置为读写。子进程通过msgsnd函数发送消息到消息队列中,父进程通过msgrcv函数从消息队列中读取消息,最后通过msgctl函数删除消息队列。
相关推荐
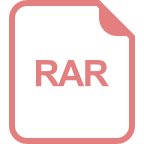
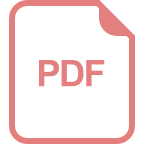
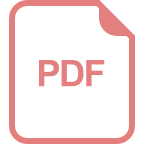













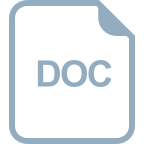
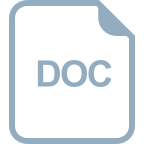
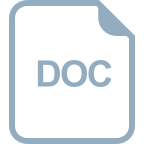