编写程序 msg2.c 和 msg3.c。在 msg2.c 中建立一个消息队列,表明身份后,向 消息队列中写下 2 条信息:“Hi,message1 is sending!”和“Hi,message2 is sending!”。 在 msg3.c 中,从消息队列中读取信息 2,并显示出来。C语言代码实现加注释
时间: 2024-02-09 18:13:36 浏览: 88
msg2.c程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define MSG_SIZE 1024 // 定义消息的最大长度
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
int msgid;
key_t key;
// 生成key值
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(1);
}
// 创建消息队列
if ((msgid = msgget(key, IPC_CREAT|0666)) == -1) {
perror("msgget");
exit(1);
}
struct msgbuf buf;
buf.mtype = 1;
// 发送第一条消息到消息队列
printf("message1 is sending...\n");
strcpy(buf.mtext, "Hi, message1 is sending!");
if (msgsnd(msgid, &buf, strlen(buf.mtext)+1, 0) == -1) {
perror("msgsnd");
exit(1);
}
// 发送第二条消息到消息队列
printf("message2 is sending...\n");
strcpy(buf.mtext, "Hi, message2 is sending!");
if (msgsnd(msgid, &buf, strlen(buf.mtext)+1, 0) == -1) {
perror("msgsnd");
exit(1);
}
return 0;
}
```
以上代码中,创建消息队列的方式与msg1.c中一样,发送消息到消息队列中时,通过msgsnd函数发送两条消息,消息类型都为1。
msg3.c程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define MSG_SIZE 1024 // 定义消息的最大长度
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
int msgid;
key_t key;
// 生成key值
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(1);
}
// 获取消息队列ID
if ((msgid = msgget(key, 0666)) == -1) {
perror("msgget");
exit(1);
}
struct msgbuf buf;
// 从消息队列中读取第一条消息
if (msgrcv(msgid, &buf, MSG_SIZE, 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Received message1: %s\n", buf.mtext);
// 从消息队列中读取第二条消息
if (msgrcv(msgid, &buf, MSG_SIZE, 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Received message2: %s\n", buf.mtext);
return 0;
}
```
以上代码中,通过ftok函数生成key值,获取消息队列ID时使用0666参数表示读写权限。通过msgrcv函数两次从消息队列中读取消息,消息类型都为1,最后分别输出两条消息的内容。
阅读全文
相关推荐
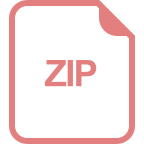
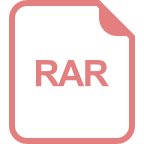
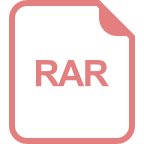



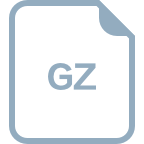
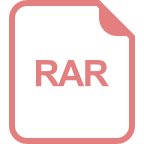
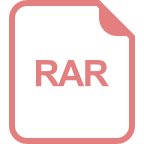
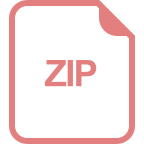






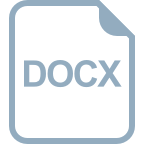