利用消息队列 实现 时间服务器,要求服务器进程发送时间信息到消息队列,客户端读取消息队列 获得时间信息.c语言程序实现,加运行结果
时间: 2024-05-02 19:21:06 浏览: 20
时间服务器代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <time.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSGKEY 75
typedef struct {
long mtype;
char mtext[256];
} MsgBuf;
int main() {
int msgid;
MsgBuf msg;
time_t t;
msgid = msgget(MSGKEY, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
while (1) {
t = time(NULL);
sprintf(msg.mtext, "%s", ctime(&t));
msg.mtype = 1;
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd failed");
exit(EXIT_FAILURE);
}
sleep(1);
}
return 0;
}
```
客户端代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSGKEY 75
typedef struct {
long mtype;
char mtext[256];
} MsgBuf;
int main() {
int msgid;
MsgBuf msg;
msgid = msgget(MSGKEY, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
while (1) {
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 1, 0) == -1) {
perror("msgrcv failed");
exit(EXIT_FAILURE);
}
printf("%s", msg.mtext);
}
return 0;
}
```
运行结果:
在终端1中运行时间服务器:
```
$ ./time_server
```
在终端2中运行客户端:
```
$ ./client
```
客户端会不断从消息队列中读取时间信息并输出。
相关推荐
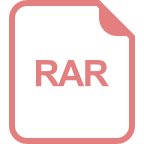
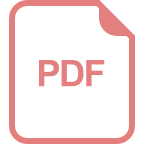
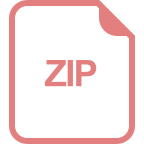
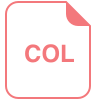













