编写一对程序send.c和recv.c,前者负责创建消息队列并发送消息(由用户输入消息),后者负责接收并打印出消息,当接收数据为“end”时结束,由后者删除该消息队列。
时间: 2024-05-12 11:15:04 浏览: 16
send.c代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAX_MSG_SIZE 1024
typedef struct {
long msg_type;
char msg_text[MAX_MSG_SIZE];
} Message;
int main() {
key_t key = ftok(".", 'a');
int msgid = msgget(key, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget failed!");
exit(EXIT_FAILURE);
}
Message message;
while (1) {
printf("Enter message: ");
fgets(message.msg_text, MAX_MSG_SIZE, stdin);
// Remove trailing newline character
size_t len = strlen(message.msg_text);
if (message.msg_text[len - 1] == '\n') {
message.msg_text[len - 1] = '\0';
}
if (strcmp(message.msg_text, "end") == 0) {
break;
}
message.msg_type = 1;
if (msgsnd(msgid, &message, MAX_MSG_SIZE, 0) == -1) {
perror("msgsnd failed!");
exit(EXIT_FAILURE);
}
}
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl(IPC_RMID) failed!");
exit(EXIT_FAILURE);
}
return 0;
}
```
recv.c代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAX_MSG_SIZE 1024
typedef struct {
long msg_type;
char msg_text[MAX_MSG_SIZE];
} Message;
int main() {
key_t key = ftok(".", 'a');
int msgid = msgget(key, 0666);
if (msgid == -1) {
perror("msgget failed!");
exit(EXIT_FAILURE);
}
Message message;
while (1) {
if (msgrcv(msgid, &message, MAX_MSG_SIZE, 0, 0) == -1) {
perror("msgrcv failed!");
exit(EXIT_FAILURE);
}
printf("Received message: %s\n", message.msg_text);
if (strcmp(message.msg_text, "end") == 0) {
break;
}
}
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl(IPC_RMID) failed!");
exit(EXIT_FAILURE);
}
return 0;
}
```
相关推荐
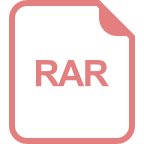
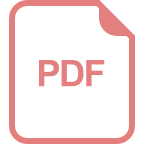
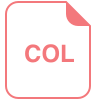
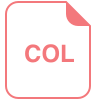
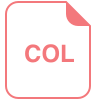
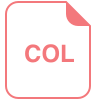
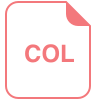









