three.js中 Cannot read properties of undefined (reading 'isGLBufferAttribute')报错
时间: 2024-12-11 14:12:16 浏览: 17
在使用three.js进行3D图形开发时,遇到“Cannot read properties of undefined (reading 'isGLBufferAttribute')”这个错误通常是由于以下几种原因导致的:
1. **属性未定义**:在访问某个属性时,该属性可能未定义或未正确初始化。
2. **版本不兼容**:使用的three.js版本与某些插件或自定义代码不兼容。
3. **资源加载问题**:某些资源(如纹理、模型等)未能正确加载,导致后续操作失败。
4. **代码错误**:代码中存在逻辑错误,导致某些对象未正确创建或初始化。
以下是一些可能的解决方法:
### 1. 检查属性初始化
确保所有需要访问的属性都已正确初始化。例如:
```javascript
let bufferAttribute;
if (bufferAttribute) {
console.log(bufferAttribute.isGLBufferAttribute);
} else {
console.error('bufferAttribute is undefined');
}
```
### 2. 版本兼容
确保使用的three.js版本与所有插件或自定义代码兼容。可以尝试更新到最新版本或回退到兼容版本。
```html
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
```
### 3. 资源加载
确保所有资源都已正确加载。可以使用`onLoad`回调来确保资源加载完成后再进行操作。
```javascript
const loader = new THREE.GLTFLoader();
loader.load('path/to/model.gltf', function(gltf) {
scene.add(gltf.scene);
// 其他操作
}, undefined, function(error) {
console.error(error);
});
```
### 4. 代码检查
仔细检查代码逻辑,确保对象创建和初始化顺序正确。例如:
```javascript
const geometry = new THREE.BufferGeometry();
// 添加属性
geometry.setAttribute('position', new THREE.Float32BufferAttribute(vertices, 3));
// 创建网格
const mesh = new THREE.Mesh(geometry, material);
scene.add(mesh);
```
通过以上步骤,可以逐步排查并解决“Cannot read properties of undefined (reading 'isGLBufferAttribute')”这个错误。
阅读全文
相关推荐
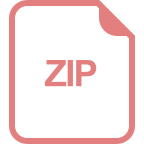
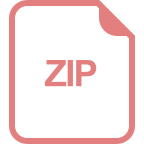
















