HTML+PHP+Mysql登录注册页面
时间: 2023-09-09 16:07:11 浏览: 35
下面是一个简单的HTML+PHP+MySQL登录注册页面的示例:
index.html:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login or Register</title>
</head>
<body>
<h1>Login or Register</h1>
<p>Please choose:</p>
<ul>
<li><a href="login.php">Login</a></li>
<li><a href="register.php">Register</a></li>
</ul>
</body>
</html>
```
login.php:
```php
<?php
session_start();
if(isset($_SESSION["username"])) {
header("Location: welcome.php");
exit();
}
require_once("config.php");
if(isset($_POST["username"]) && isset($_POST["password"])) {
$username = $_POST["username"];
$password = md5($_POST["password"]);
$sql = "SELECT * FROM users WHERE username='$username' AND password='$password'";
$result = mysqli_query($conn, $sql);
if(mysqli_num_rows($result) == 1) {
$row = mysqli_fetch_assoc($result);
$_SESSION["username"] = $row["username"];
header("Location: welcome.php");
exit();
} else {
$error = "Invalid username or password";
}
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<?php if(isset($error)) echo "<p style='color:red;'>$error</p>"; ?>
<form method="post" action="">
<p>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
</p>
<p>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
</p>
<p>
<button type="submit">Login</button>
</p>
</form>
</body>
</html>
```
register.php:
```php
<?php
require_once("config.php");
if(isset($_POST["username"]) && isset($_POST["password"])) {
$username = $_POST["username"];
$password = md5($_POST["password"]);
$sql = "INSERT INTO users (username, password) VALUES ('$username', '$password')";
if(mysqli_query($conn, $sql)) {
header("Location: login.php");
exit();
} else {
$error = "Registration failed";
}
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Register</title>
</head>
<body>
<h1>Register</h1>
<?php if(isset($error)) echo "<p style='color:red;'>$error</p>"; ?>
<form method="post" action="">
<p>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
</p>
<p>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
</p>
<p>
<button type="submit">Register</button>
</p>
</form>
</body>
</html>
```
config.php:
```php
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "mydb";
$conn = mysqli_connect($servername, $username, $password, $dbname);
if(!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
?>
```
注意:这只是一个简单的示例,实际应用中需要进行更多的安全性检查和数据验证。
相关推荐
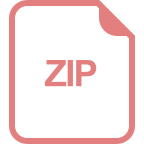














