一条线段如何向两边 扩大 c++代码
时间: 2024-01-31 16:02:34 浏览: 22
以下是一个简单的C++函数,可以将一条线段向两边扩大指定的距离:
```c++
#include <iostream>
#include <cmath>
using namespace std;
struct Point {
double x, y;
};
struct Line {
Point start, end;
};
// 计算两点之间的距离
double distance(Point p1, Point p2) {
return sqrt(pow(p2.x - p1.x, 2) + pow(p2.y - p1.y, 2));
}
// 计算线段的斜率
double slope(Line line) {
return (line.end.y - line.start.y) / (line.end.x - line.start.x);
}
// 计算线段的长度
double length(Line line) {
return distance(line.start, line.end);
}
// 将线段向两边扩大指定的距离
void expand(Line& line, double distance) {
double dx = (line.end.x - line.start.x) * distance / length(line);
double dy = (line.end.y - line.start.y) * distance / length(line);
Point left = {line.start.x - dy, line.start.y + dx};
Point right = {line.end.x - dy, line.end.y + dx};
line.start = left;
line.end = right;
}
int main() {
Line line = {{0, 0}, {3, 4}};
double distance = 1;
cout << "Original line: (" << line.start.x << "," << line.start.y << ") to (" << line.end.x << "," << line.end.y << ")" << endl;
expand(line, distance);
cout << "Expanded line: (" << line.start.x << "," << line.start.y << ") to (" << line.end.x << "," << line.end.y << ")" << endl;
return 0;
}
```
这个函数采用结构体表示点和线段,使用欧几里得距离计算点之间的距离,使用勾股定理计算线段长度,并使用向量的方法将线段向两边扩大指定的距离。
相关推荐
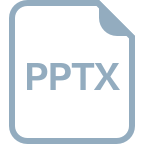














