c语言文件IO在linux实现cp命令复制功能
时间: 2023-08-30 17:06:17 浏览: 221
在Linux中,可以使用文件IO的方式来实现cp命令的复制功能。具体步骤如下:
1. 打开源文件和目标文件,可以使用open()函数,如下所示:
```
int src_fd = open("source_file", O_RDONLY);
int dest_fd = open("destination_file", O_WRONLY|O_CREAT, S_IRUSR|S_IWUSR);
```
其中,第一个参数为文件名,第二个参数为打开文件的模式,第三个参数为文件权限。
2. 读取源文件的内容并写入目标文件中,可以使用read()和write()函数,如下所示:
```
char buffer[1024];
int read_size = 0;
while((read_size = read(src_fd, buffer, sizeof(buffer))) > 0) {
write(dest_fd, buffer, read_size);
}
```
其中,第一个参数为文件描述符,第二个参数为读取或写入的缓冲区,第三个参数为缓冲区的大小。
3. 关闭源文件和目标文件,可以使用close()函数,如下所示:
```
close(src_fd);
close(dest_fd);
```
完整代码如下所示:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
int main(int argc, char *argv[]) {
if(argc != 3) {
printf("Usage: %s <source_file> <destination_file>\n", argv[0]);
return 1;
}
int src_fd = open(argv[1], O_RDONLY);
if(src_fd < 0) {
printf("Failed to open source file: %s\n", argv[1]);
return 1;
}
int dest_fd = open(argv[2], O_WRONLY|O_CREAT, S_IRUSR|S_IWUSR);
if(dest_fd < 0) {
printf("Failed to open destination file: %s\n", argv[2]);
close(src_fd);
return 1;
}
char buffer[1024];
int read_size = 0;
while((read_size = read(src_fd, buffer, sizeof(buffer))) > 0) {
write(dest_fd, buffer, read_size);
}
close(src_fd);
close(dest_fd);
return 0;
}
```
将上面的代码保存为cp.c文件,然后使用gcc编译即可生成可执行文件cp:
```
gcc cp.c -o cp
```
使用方法:
```
./cp source_file destination_file
```
其中,source_file为源文件名,destination_file为目标文件名。
阅读全文
相关推荐












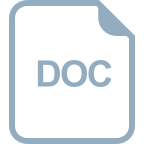




