# import pandas as pd # df = pd.read_excel('sheet.xlsx', engine='openpyxl') # new_df = df.query('id == "220"') # writer = pd.ExcelWriter('new_excel_file.xlsx') # new_df.to_excel(writer, sheet_name='New_Sheet', index=False) # writer.save() import openpyxl # 读取Excel文件 workbook = openpyxl.load_workbook('sheet.xlsx') # 选择需要操作的sheet页 worksheet = workbook['Sheet'] # 根据筛选条件获取满足条件的数据,其中,min_row=2表示从第二行开始读取数据,因为第一行通常是表头; # max_row=worksheet.max_row表示读取Excel文件中所有的行数据;values_only=True表示只读取单元格中的值,而不包括格式等其他元素。 new_data = [] for row in worksheet.iter_rows(min_row=2, max_row=worksheet.max_row, values_only=True): if row[2] == '220': new_data.append(row) # 创建新的sheet页并写入数据 new_worksheet = workbook.create_sheet('New Sheet') for row in new_data: new_worksheet.append(row) # 保存Excel文件 workbook.save('sheet.xlsx')报zipfile.BadZipFile: File is not a zip file
时间: 2024-04-05 09:31:47 浏览: 26
这个错误通常是因为你的 Excel 文件已经被其他程序打开了,或者文件损坏导致的。你可以尝试先关闭 Excel 文件,然后重新运行代码,或者尝试使用另一个 Excel 文件来测试代码是否正常运行。如果问题仍然存在,你可以尝试使用 openpyxl 库提供的 load_workbook() 函数的 data_only 参数,将其设置为 True,这样可以确保仅读取单元格中的值,而不包括公式等其他元素。
相关问题
import pandas as pddf = pd.read_excel('path/to/file.xlsx')
这段代码使用 Pandas 库中的 `read_excel` 函数来读取 Excel 文件并将其存储为 DataFrame 对象,代码中的 `path/to/file.xlsx` 应该被替换为实际文件路径。如果文件位于当前工作目录中,则可以直接使用文件名而不需要路径。例如,如果文件名为 `data.xlsx`,则可以使用以下代码:
```
import pandas as pd
df = pd.read_excel('data.xlsx')
```
这将读取名为 `data.xlsx` 的文件并将其存储为 DataFrame 对象 `df`。
import pandas as pd df = pd.read_excel('京津冀红色.xlsx') print(df)
这是一个使用 Pandas 库读取 Excel 文件并打印数据的 Python 代码。`import pandas as pd` 是导入 Pandas 库并给它起了一个别名 `pd`。`pd.read_excel('京津冀红色.xlsx')` 是读取名为 `京津冀红色.xlsx` 的 Excel 文件并将数据存储在一个名为 `df` 的 Pandas DataFrame 对象中。`print(df)` 是将 DataFrame 对象的内容打印出来。您需要告诉我您对这段代码的具体问题,我才能为您提供更详细的解答。
相关推荐
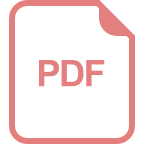
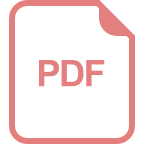
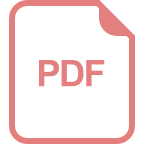













