推箱子小游戏java代码
时间: 2023-08-28 07:06:20 浏览: 41
下面是一个简单的推箱子小游戏的 Java 代码,你可以根据自己的需求进行修改和完善:
```
import java.util.Scanner;
public class PushBoxGame {
// 地图大小
private static final int ROW = 6;
private static final int COL = 6;
// 地图数组
private static char[][] map = new char[][]{
{'#', '#', '#', '#', '#', '#'},
{'#', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', '@', ' ', ' ', '#'},
{'#', ' ', 'O', ' ', ' ', '#'},
{'#', '#', '#', '#', '#', '#'}
};
// 玩家坐标
private static int playerRow = 3;
private static int playerCol = 2;
// 箱子坐标
private static int boxRow = 4;
private static int boxCol = 2;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
// 打印地图
printMap();
// 判断是否胜利
if (isWin()) {
System.out.println("恭喜你,胜利啦!");
break;
}
// 获取玩家输入
System.out.print("请输入操作(w:上 s:下 a:左 d:右):");
String input = scanner.nextLine();
// 移动玩家
movePlayer(input);
// 移动箱子
moveBox(input);
}
}
// 打印地图
private static void printMap() {
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
System.out.print(map[i][j]);
}
System.out.println();
}
}
// 移动玩家
private static void movePlayer(String input) {
switch (input) {
case "w":
if (map[playerRow - 1][playerCol] != '#') {
map[playerRow][playerCol] = ' ';
playerRow--;
map[playerRow][playerCol] = '@';
}
break;
case "s":
if (map[playerRow + 1][playerCol] != '#') {
map[playerRow][playerCol] = ' ';
playerRow++;
map[playerRow][playerCol] = '@';
}
break;
case "a":
if (map[playerRow][playerCol - 1] != '#') {
map[playerRow][playerCol] = ' ';
playerCol--;
map[playerRow][playerCol] = '@';
}
break;
case "d":
if (map[playerRow][playerCol + 1] != '#') {
map[playerRow][playerCol] = ' ';
playerCol++;
map[playerRow][playerCol] = '@';
}
break;
}
}
// 移动箱子
private static void moveBox(String input) {
switch (input) {
case "w":
if (playerRow - 1 == boxRow && playerCol == boxCol && map[boxRow - 1][boxCol] != '#') {
map[boxRow][boxCol] = ' ';
boxRow--;
map[boxRow][boxCol] = 'O';
}
break;
case "s":
if (playerRow + 1 == boxRow && playerCol == boxCol && map[boxRow + 1][boxCol] != '#') {
map[boxRow][boxCol] = ' ';
boxRow++;
map[boxRow][boxCol] = 'O';
}
break;
case "a":
if (playerRow == boxRow && playerCol - 1 == boxCol && map[boxRow][boxCol - 1] != '#') {
map[boxRow][boxCol] = ' ';
boxCol--;
map[boxRow][boxCol] = 'O';
}
break;
case "d":
if (playerRow == boxRow && playerCol + 1 == boxCol && map[boxRow][boxCol + 1] != '#') {
map[boxRow][boxCol] = ' ';
boxCol++;
map[boxRow][boxCol] = 'O';
}
break;
}
}
// 判断是否胜利
private static boolean isWin() {
return map[boxRow - 1][boxCol] == '$' && map[boxRow + 1][boxCol] == '$'
&& map[boxRow][boxCol - 1] == '$' && map[boxRow][boxCol + 1] == '$';
}
}
```
在这个代码中,使用了一个二维字符数组来表示地图,其中 '#' 表示墙,' ' 表示空地,'@' 表示玩家,'O' 表示箱子。通过判断玩家的输入来移动玩家和箱子,并且在移动过程中需要判断是否能够移动,如果不能移动,则不执行移动操作。在每次移动后需要判断是否胜利,如果箱子已经全部推到了目标位置,则游戏胜利。
相关推荐
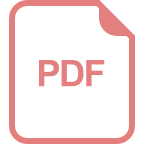
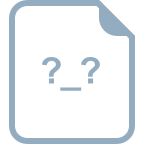
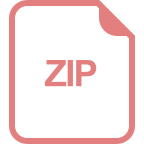












