从网上获取任意英文文本素材,分词后统计词频,将高频词用第三方库wordcloud可视化为词云。
时间: 2023-06-09 21:02:29 浏览: 143
好的,这个问题很有趣!可以使用Python的一些第三方库来实现这个任务。首先,我们可以使用requests库或者urllib库来获取英文文本素材。接下来,可以使用nltk库或者jieba库等工具进行分词,分别可以处理英文和中文文本。然后,可以使用collections库来统计词频并找到高频词。最后,使用wordcloud库将高频词可视化为词云。如果你需要更详细的操作步骤和代码示例,请告诉我。
相关问题
打卡桌面中的22012515文件夹,读取其中的文档,将高频词用wordcloud可视化为词云,用python语言
好的,首先需要安装一些必要的库,包括`jieba`用于中文分词和`wordcloud`用于生成词云图。
```python
!pip install jieba
!pip install wordcloud
```
然后我们可以开始读取文件并进行分词,计算高频词。
```python
import os
import jieba
from collections import Counter
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文件夹中所有的文档
folder_path = '22012515'
file_list = os.listdir(folder_path)
doc_list = []
for file_name in file_list:
file_path = os.path.join(folder_path, file_name)
with open(file_path, 'r', encoding='utf-8') as f:
doc_list.append(f.read())
# 对所有文档进行分词,统计高频词
word_count = Counter()
for doc in doc_list:
words = jieba.cut(doc)
for word in words:
if len(word) > 1: # 只统计长度大于1的词语
word_count[word] += 1
# 获取前50个高频词
top50_words = word_count.most_common(50)
```
接下来,我们可以将高频词可视化为词云。
```python
# 生成词云图
wordcloud = WordCloud(font_path='simhei.ttf', background_color='white', width=800, height=600)
wordcloud.generate_from_frequencies(dict(top50_words))
# 显示词云图
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
这样就可以生成一个高频词的词云图了。你可以根据需要调整字体、背景颜色、词云图大小等参数。
完整的代码如下:
```python
import os
import jieba
from collections import Counter
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文件夹中所有的文档
folder_path = '22012515'
file_list = os.listdir(folder_path)
doc_list = []
for file_name in file_list:
file_path = os.path.join(folder_path, file_name)
with open(file_path, 'r', encoding='utf-8') as f:
doc_list.append(f.read())
# 对所有文档进行分词,统计高频词
word_count = Counter()
for doc in doc_list:
words = jieba.cut(doc)
for word in words:
if len(word) > 1: # 只统计长度大于1的词语
word_count[word] += 1
# 获取前50个高频词
top50_words = word_count.most_common(50)
# 生成词云图
wordcloud = WordCloud(font_path='simhei.ttf', background_color='white', width=800, height=600)
wordcloud.generate_from_frequencies(dict(top50_words))
# 显示词云图
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
编写Python程序,从网上获取中文时事文本素材(保存为txt文本),利用第三方库jieba分词后统计词频并输出(从高到低)。
以下是示例代码:
```python
import requests
import jieba
from collections import Counter
# 从网上获取中文时事文本素材,这里以新浪新闻为例
url = 'https://news.sina.com.cn/china/'
response = requests.get(url)
text = response.text
# 保存为txt文本
with open('news.txt', 'w', encoding='utf-8') as f:
f.write(text)
# 读取文本内容并进行分词
with open('news.txt', 'r', encoding='utf-8') as f:
text = f.read()
words = jieba.cut(text)
# 统计词频并输出结果
word_counts = Counter(words)
top_words = word_counts.most_common(20) # 只输出出现频率最高的前20个词语
for word, count in top_words:
print(f'{word}\t{count}')
```
解释一下代码的各部分:
1. 使用requests库获取中文时事文本素材,并保存为txt文本。
2. 使用jieba库进行分词。
3. 使用collections库的Counter类统计词频。
4. 输出出现频率最高的前20个词语及其出现次数。
需要注意的是,jieba库需要提前安装。可以使用以下命令进行安装:
```
pip install jieba
```
阅读全文
相关推荐
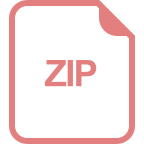
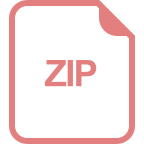
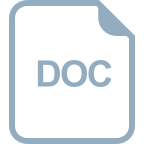












