c语言稀疏矩阵运算器使用十字链表算法
时间: 2023-07-26 07:40:26 浏览: 115
实现稀疏矩阵运算器可以使用十字链表算法,具体步骤如下:
1. 定义稀疏矩阵的节点类型:
```
typedef struct Node {
int row; // 行号
int col; // 列号
int value; // 值
struct Node *right, *down; // 指向右边和下面节点的指针
} Node, *PNode;
```
2. 定义稀疏矩阵类型:
```
typedef struct {
int rows; // 行数
int cols; // 列数
PNode *row_heads; // 每行的头节点
PNode *col_heads; // 每列的头节点
} SparseMatrix, *PSparseMatrix;
```
3. 初始化稀疏矩阵:
```
PSparseMatrix initSparseMatrix(int rows, int cols) {
PSparseMatrix matrix = (PSparseMatrix) malloc(sizeof(SparseMatrix));
matrix->rows = rows;
matrix->cols = cols;
matrix->row_heads = (PNode *) malloc(sizeof(PNode) * rows);
matrix->col_heads = (PNode *) malloc(sizeof(PNode) * cols);
for (int i = 0; i < rows; i++) {
matrix->row_heads[i] = (PNode) malloc(sizeof(Node));
matrix->row_heads[i]->right = NULL;
matrix->row_heads[i]->down = NULL;
}
for (int i = 0; i < cols; i++) {
matrix->col_heads[i] = (PNode) malloc(sizeof(Node));
matrix->col_heads[i]->right = NULL;
matrix->col_heads[i]->down = NULL;
}
return matrix;
}
```
4. 插入稀疏矩阵节点:
```
void insertSparseMatrixNode(PSparseMatrix matrix, int row, int col, int value) {
PNode node = (PNode) malloc(sizeof(Node));
node->row = row;
node->col = col;
node->value = value;
PNode row_head = matrix->row_heads[row];
PNode col_head = matrix->col_heads[col];
PNode row_prev = row_head, col_prev = col_head;
while (row_prev->right && row_prev->right->col < col) {
row_prev = row_prev->right;
}
while (col_prev->down && col_prev->down->row < row) {
col_prev = col_prev->down;
}
node->right = row_prev->right;
node->down = col_prev->down;
row_prev->right = node;
col_prev->down = node;
}
```
5. 稀疏矩阵相加:
```
PSparseMatrix addSparseMatrix(PSparseMatrix matrix1, PSparseMatrix matrix2) {
PSparseMatrix result = initSparseMatrix(matrix1->rows, matrix1->cols);
for (int i = 0; i < matrix1->rows; i++) {
PNode node1 = matrix1->row_heads[i]->right;
PNode node2 = matrix2->row_heads[i]->right;
PNode result_prev = result->row_heads[i];
while (node1 || node2) {
PNode node;
if (node1 && (!node2 || node1->col < node2->col)) {
node = (PNode) malloc(sizeof(Node));
node->row = i;
node->col = node1->col;
node->value = node1->value;
node1 = node1->right;
} else if (node2 && (!node1 || node2->col < node1->col)) {
node = (PNode) malloc(sizeof(Node));
node->row = i;
node->col = node2->col;
node->value = node2->value;
node2 = node2->right;
} else {
node = (PNode) malloc(sizeof(Node));
node->row = i;
node->col = node1->col;
node->value = node1->value + node2->value;
node1 = node1->right;
node2 = node2->right;
}
node->right = result_prev->right;
result_prev->right = node;
result_prev = node;
}
}
return result;
}
```
6. 打印稀疏矩阵:
```
void printSparseMatrix(PSparseMatrix matrix) {
for (int i = 0; i < matrix->rows; i++) {
PNode node = matrix->row_heads[i]->right;
for (int j = 0; j < matrix->cols; j++) {
if (node && node->col == j) {
printf("%d ", node->value);
node = node->right;
} else {
printf("0 ");
}
}
printf("\n");
}
}
```
以上就是使用十字链表算法实现稀疏矩阵运算器的基本步骤,可以根据需要进行修改和扩展。
阅读全文
相关推荐
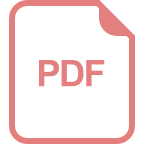
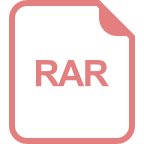
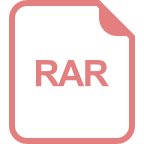
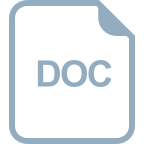
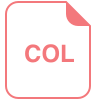
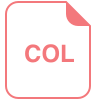
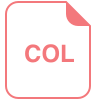
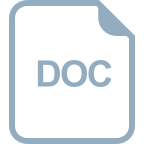
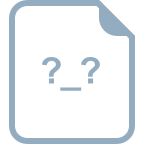
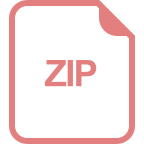
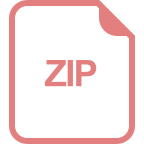