《1》 编写函数int getDepth(BiTNode *T),计算二叉树T的深度
时间: 2024-01-22 20:17:18 浏览: 98
可以使用递归的方式来计算二叉树的深度,具体实现如下:
```c++
int getDepth(BiTNode *T) {
if (T == nullptr) { // 如果节点为空,返回0
return 0;
}
int leftDepth = getDepth(T->lchild); // 计算左子树的深度
int rightDepth = getDepth(T->rchild); // 计算右子树的深度
return 1 + (leftDepth > rightDepth ? leftDepth : rightDepth); // 返回较大值加上当前节点的深度1
}
```
其中,对于一个节点的深度,可以定义为其所在的层数,根节点的深度为1。递归实现时,先判断当前节点是否为空,如果是,则返回0;否则,分别计算其左子树和右子树的深度,并取较大值,最后加上当前节点的深度1,即可得到整个二叉树的深度。
相关问题
《1》编写函数int getDepth(BiTNode *T),计算二叉树T的深度
可以使用递归来实现计算二叉树深度的功能。具体实现可以参考下面的代码:
```
int getDepth(BiTNode *T)
{
if(T == NULL) return 0; // 空树深度为0
int leftDepth = getDepth(T->lchild); // 计算左子树深度
int rightDepth = getDepth(T->rchild); // 计算右子树深度
return (leftDepth > rightDepth) ? (leftDepth + 1) : (rightDepth + 1); // 返回深度
}
```
在递归过程中,如果当前节点为空,则返回0;否则,分别计算左子树和右子树的深度,并返回二者中较大的一个再加1。
编写函数int getDepth(BiTNode *T),计算二叉树T的深度,并给出完整代码
以下是计算二叉树深度的完整代码,其中getDepth函数返回二叉树的深度:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct BiTNode {
int data;
struct BiTNode *lchild, *rchild;
} BiTNode, *BiTree;
int getDepth(BiTree T);
int main() {
// 构造二叉树
BiTree T = (BiTree) malloc(sizeof(BiTNode));
T->data = 1;
T->lchild = (BiTree) malloc(sizeof(BiTNode));
T->lchild->data = 2;
T->rchild = (BiTree) malloc(sizeof(BiTNode));
T->rchild->data = 3;
T->lchild->lchild = (BiTree) malloc(sizeof(BiTNode));
T->lchild->lchild->data = 4;
T->lchild->rchild = (BiTree) malloc(sizeof(BiTNode));
T->lchild->rchild->data = 5;
printf("二叉树的深度为:%d\n", getDepth(T));
return 0;
}
int getDepth(BiTree T) {
if (T == NULL) {
return 0;
} else {
int leftDepth = getDepth(T->lchild);
int rightDepth = getDepth(T->rchild);
return (leftDepth > rightDepth) ? (leftDepth + 1) : (rightDepth + 1);
}
}
```
在上面的代码中,我们使用了递归的思想来计算二叉树的深度。如果当前节点为空,则返回0;否则,分别计算左子树和右子树的深度,并取较大值加1作为当前节点的深度。最终的二叉树深度即为根节点的深度。
阅读全文
相关推荐
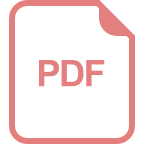



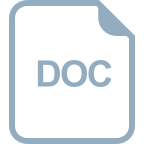


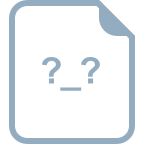
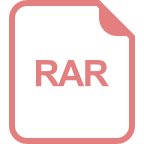
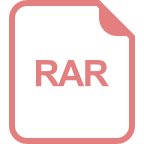