c语言 构造利用二叉链表存储表示的二叉树 利用先序遍历创建一个二叉树 递归计算二叉树的深度和叶子结点的个数。
时间: 2023-12-01 17:57:15 浏览: 42
好的,下面是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树的结构体
typedef struct BiTNode {
char data;
struct BiTNode *lchild, *rchild;
} BiTNode, *BiTree;
// 先序遍历创建二叉树
void CreateBiTree(BiTree *T) {
char c;
scanf("%c", &c);
if (c == ' ') {
*T = NULL;
} else {
*T = (BiTree)malloc(sizeof(BiTNode));
(*T)->data = c;
CreateBiTree(&(*T)->lchild);
CreateBiTree(&(*T)->rchild);
}
}
// 递归计算二叉树深度
int GetDepth(BiTree T) {
if (!T) {
return 0;
} else {
int left_depth = GetDepth(T->lchild);
int right_depth = GetDepth(T->rchild);
return (left_depth > right_depth ? left_depth : right_depth) + 1;
}
}
// 递归计算叶子节点个数
int GetLeafCount(BiTree T) {
if (!T) {
return 0;
} else if (!T->lchild && !T->rchild) {
return 1;
} else {
return GetLeafCount(T->lchild) + GetLeafCount(T->rchild);
}
}
int main() {
BiTree T;
printf("请按先序遍历的顺序输入二叉树(空格表示空节点):\n");
CreateBiTree(&T);
printf("二叉树的深度为:%d\n", GetDepth(T));
printf("叶子节点的个数为:%d\n", GetLeafCount(T));
return 0;
}
```
以上代码中,`BiTNode`是二叉树的结构体,包括数据域`data`和左右孩子指针`lchild`和`rchild`。`CreateBiTree`函数用于先序遍历创建二叉树。`GetDepth`函数用于递归计算二叉树的深度。`GetLeafCount`函数用于递归计算叶子节点个数。在`main`函数中,先调用`CreateBiTree`函数创建二叉树,然后调用`GetDepth`和`GetLeafCount`函数计算深度和叶子节点个数并输出结果。
相关推荐
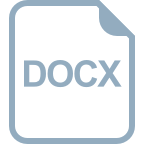
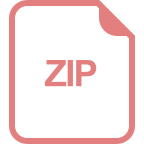














