C++从键盘输入字符串(最多30个),之后再输入单个字符,将字符串s中所有ch字符删除掉,然后输出新的字符串。
时间: 2024-02-12 20:02:53 浏览: 32
好的,可以通过以下代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s;
char ch;
getline(cin, s); // 从键盘输入字符串
cin >> ch; // 输入要删除的字符
for (int i = 0; i < s.length(); i++) {
if (s[i] != ch) {
cout << s[i]; // 输出不是要删除的字符的字符
}
}
cout << endl;
return 0;
}
```
代码中用 `getline(cin, s)` 从键盘输入字符串,而不是使用 `cin >> s`,是因为前者可以读取包括空格在内的一整行字符串,而后者只能读取一个单词。然后再通过 `cin >> ch` 输入要删除的字符。接着用循环遍历字符串中的每个字符,如果不是要删除的字符,就输出它。最后记得换行并返回0。
相关问题
从键盘输入字符串s(最多30个)和单个字符ch,将字符串s中所有ch字符删除掉,然后输出新的字符串。c++
以下是C++代码实现:
```c++
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s;
char ch;
getline(cin, s); // 输入字符串s
cin >> ch; // 输入要删除的字符ch
for(int i = 0; i < s.length(); i++)
{
if(s[i] != ch) // 如果当前字符不是要删除的字符ch
{
cout << s[i]; // 输出当前字符
}
}
cout << endl;
return 0;
}
```
首先使用`getline()`函数输入字符串`s`,然后使用`cin`输入要删除的字符`ch`。接着使用一个循环遍历字符串`s`中的每个字符,如果该字符不等于要删除的字符`ch`,则输出该字符。循环结束后,输出一个换行符,程序结束。
C++如何判断单个字符是否在字符串中
可以使用标准库函数 `strchr()` 来判断单个字符是否在字符串中。
`strchr()` 函数的原型如下:
```c
char *strchr(const char *str, int c);
```
其中,第一个参数 `str` 是要查找的字符串;第二个参数 `c` 是要查找的字符。
函数返回值是一个指向第一次出现字符的指针,如果没有找到这个字符,则返回 `NULL`。
因此,我们可以使用以下代码来判断一个字符串是否包含某个字符:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello World";
char ch = 'o';
char *result = strchr(str, ch);
if (result != NULL) {
printf("%c is found in %s\n", ch, str);
} else {
printf("%c is not found in %s\n", ch, str);
}
return 0;
}
```
输出结果为:
```
o is found in Hello World
```
相关推荐
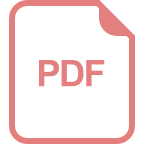
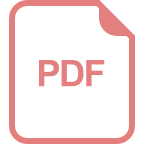












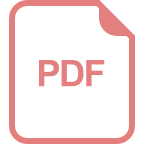