以生产者/消费者模型为依据,在 linux 环境下创建一个控制台进程,在该进 程中创建 n 个线程模拟生产者和消费者,实现进程(线程)的同步与互斥。
时间: 2024-02-23 11:58:06 浏览: 56
好的,这是一个关于多线程同步与互斥的问题。我们可以使用 pthread 库来创建线程。下面是一个实现生产者/消费者模型的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 5 // 缓冲区大小
#define PRODUCER_NUM 3 // 生产者数量
#define CONSUMER_NUM 2 // 消费者数量
int buffer[BUFFER_SIZE]; // 缓冲区
int in = 0; // 生产者放置产品的位置
int out = 0; // 消费者取产品的位置
int count = 0; // 缓冲区中产品的数量
pthread_mutex_t mutex; // 互斥量
pthread_cond_t cond_empty; // 缓冲区为空的条件变量
pthread_cond_t cond_full; // 缓冲区为满的条件变量
void *producer(void *arg)
{
int id = *(int *)arg;
while (1) {
// 生产者获取互斥量
pthread_mutex_lock(&mutex);
// 如果缓冲区已满,等待条件变量 cond_full
while (count == BUFFER_SIZE) {
printf("producer %d: buffer is full, wait...\n", id);
pthread_cond_wait(&cond_full, &mutex);
}
// 生产产品
buffer[in] = 1;
printf("producer %d: produce an item, buffer[%d]=%d\n", id, in, buffer[in]);
in = (in + 1) % BUFFER_SIZE;
count++;
// 发送条件变量 cond_empty
pthread_cond_signal(&cond_empty);
// 释放互斥量
pthread_mutex_unlock(&mutex);
// 休眠一段时间
sleep(rand() % 3);
}
return NULL;
}
void *consumer(void *arg)
{
int id = *(int *)arg;
while (1) {
// 消费者获取互斥量
pthread_mutex_lock(&mutex);
// 如果缓冲区为空,等待条件变量 cond_empty
while (count == 0) {
printf("consumer %d: buffer is empty, wait...\n", id);
pthread_cond_wait(&cond_empty, &mutex);
}
// 消费产品
int item = buffer[out];
buffer[out] = 0;
printf("consumer %d: consume an item, buffer[%d]=%d\n", id, out, item);
out = (out + 1) % BUFFER_SIZE;
count--;
// 发送条件变量 cond_full
pthread_cond_signal(&cond_full);
// 释放互斥量
pthread_mutex_unlock(&mutex);
// 休眠一段时间
sleep(rand() % 3);
}
return NULL;
}
int main()
{
int i;
pthread_t tid;
int id[PRODUCER_NUM + CONSUMER_NUM];
// 初始化互斥量和条件变量
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond_empty, NULL);
pthread_cond_init(&cond_full, NULL);
// 创建生产者线程
for (i = 0; i < PRODUCER_NUM; i++) {
id[i] = i;
pthread_create(&tid, NULL, producer, &id[i]);
}
// 创建消费者线程
for (i = 0; i < CONSUMER_NUM; i++) {
id[i + PRODUCER_NUM] = i;
pthread_create(&tid, NULL, consumer, &id[i + PRODUCER_NUM]);
}
// 等待所有线程结束
for (i = 0; i < PRODUCER_NUM + CONSUMER_NUM; i++) {
pthread_join(tid, NULL);
}
// 销毁互斥量和条件变量
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond_empty);
pthread_cond_destroy(&cond_full);
return 0;
}
```
在这个示例中,我们使用了一个长度为 BUFFER_SIZE 的缓冲区来模拟生产者和消费者之间的交互。生产者在缓冲区中生产产品,消费者从缓冲区中消费产品。在生产者和消费者之间,我们使用了互斥量来保证同时只有一个线程可以访问缓冲区。同时,我们还使用了两个条件变量,一个表示缓冲区为空,一个表示缓冲区为满。当缓冲区为空时,消费者等待条件变量 cond_empty,当缓冲区为满时,生产者等待条件变量 cond_full。
希望这个示例能够帮助你理解多线程同步与互斥的概念。
阅读全文
相关推荐
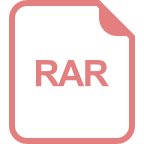
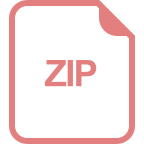
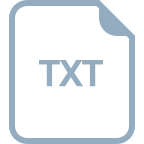
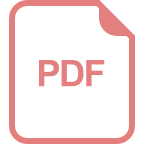
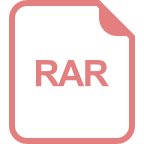
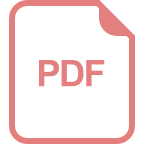
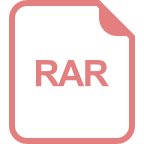
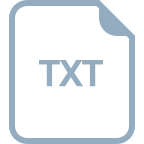
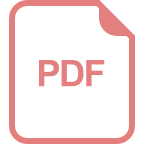
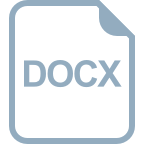
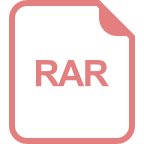
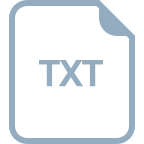
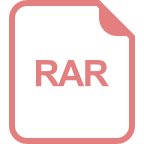
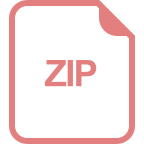
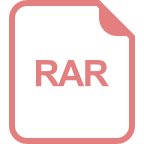
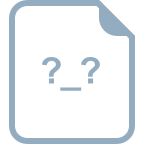
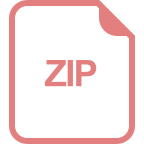