sqlite3 连接池 C
时间: 2023-07-29 11:12:26 浏览: 165
以下是一个基于 C 语言的 SQLite3 连接池示例:
```c
#include <sqlite3.h>
#include <stdlib.h>
#include <stdio.h>
#include <stdbool.h>
#include <pthread.h>
typedef struct {
sqlite3 *conn;
bool in_use;
} Connection;
typedef struct {
int max_connections;
Connection *connections;
pthread_mutex_t lock;
} ConnectionPool;
void init_pool(ConnectionPool *pool, int max_connections) {
pool->max_connections = max_connections;
pool->connections = (Connection*) malloc(max_connections * sizeof(Connection));
pthread_mutex_init(&pool->lock, NULL);
for(int i = 0; i < max_connections; i++) {
pool->connections[i].conn = NULL;
pool->connections[i].in_use = false;
}
}
void destroy_pool(ConnectionPool *pool) {
for(int i = 0; i < pool->max_connections; i++) {
if(pool->connections[i].conn != NULL) {
sqlite3_close(pool->connections[i].conn);
}
}
free(pool->connections);
pthread_mutex_destroy(&pool->lock);
}
sqlite3* get_connection(ConnectionPool *pool) {
pthread_mutex_lock(&pool->lock);
for(int i = 0; i < pool->max_connections; i++) {
if(!pool->connections[i].in_use) {
if(pool->connections[i].conn == NULL) {
int rc = sqlite3_open("mydatabase.db", &pool->connections[i].conn);
if(rc != SQLITE_OK) {
fprintf(stderr, "Cannot open database: %s\n", sqlite3_errmsg(pool->connections[i].conn));
sqlite3_close(pool->connections[i].conn);
pool->connections[i].conn = NULL;
continue;
}
}
pool->connections[i].in_use = true;
pthread_mutex_unlock(&pool->lock);
return pool->connections[i].conn;
}
}
pthread_mutex_unlock(&pool->lock);
return NULL;
}
void release_connection(ConnectionPool *pool, sqlite3 *conn) {
pthread_mutex_lock(&pool->lock);
for(int i = 0; i < pool->max_connections; i++) {
if(pool->connections[i].conn == conn) {
pool->connections[i].in_use = false;
break;
}
}
pthread_mutex_unlock(&pool->lock);
}
```
在上述示例中,我们定义了一个 Connection 结构体,表示一个连接,包含一个 sqlite3 指针和一个布尔值,用于标记该连接是否正在被使用。我们还定义了一个 ConnectionPool 结构体,表示连接池,包含一个最大连接数、一个 Connection 数组和一个互斥锁。在 init_pool 函数中,我们初始化连接池,分配 Connection 数组空间,初始化锁和 Connection 结构体。在 destroy_pool 函数中,我们关闭连接池中所有的连接,并释放 Connection 数组空间和锁。在 get_connection 函数中,我们使用互斥锁来保证线程安全,遍历 Connection 数组,寻找可用的连接。如果找到了一个未被使用的连接,我们检查该连接是否为 NULL,如果是,我们打开数据库并将连接保存到 Connection 结构体中。然后,我们将 Connection 结构体中的 in_use 字段设置为 true,释放锁并返回连接。如果遍历完 Connection 数组仍未找到可用的连接,我们释放锁并返回 NULL。在 release_connection 函数中,我们使用互斥锁来保证线程安全,遍历 Connection 数组,找到与指定连接相同的 Connection 结构体,并将该结构体中的 in_use 字段设置为 false,释放锁。该示例仅供参考,实际使用时需要根据具体情况进行修改和优化。
阅读全文
相关推荐
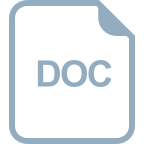
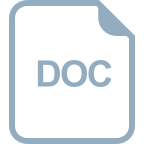
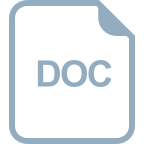

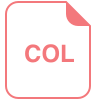

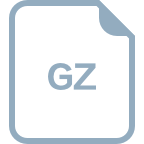
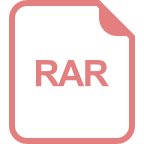
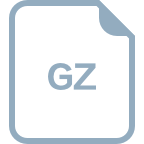
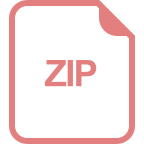
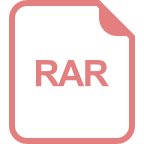
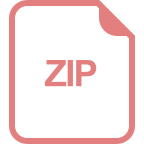
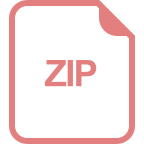
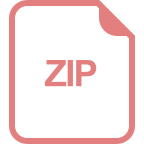
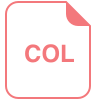
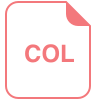

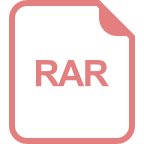
