用java实现:最长公共子串的长度 描述:给定两个字符串str1和str2,输出两个字符串的最长公共子串的长度(假设str1和str2的最长公共子串存在且唯一)。
时间: 2024-01-07 14:43:47 浏览: 80
以下是用Java实现最长公共子串长度的示例代码:
```java
public class LongestCommonSubstring {
public static int longestCommonSubstring(String str1, String str2) {
int m = str1.length();
int n = str2.length();
int maxLength = 0;
int[][] dp = new int[m+1][n+1];
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (str1.charAt(i-1) == str2.charAt(j-1)) {
dp[i][j] = dp[i-1][j-1] + 1;
maxLength = Math.max(maxLength, dp[i][j]);
}
}
}
return maxLength;
}
public static void main(String[] args) {
String str1 = "abcdxyz";
String str2 = "xyzabcd";
int maxLength = longestCommonSubstring(str1, str2);
System.out.println("The length of longest common substring is " + maxLength);
}
}
```
以上代码使用动态规划算法来解决该问题。通过定义一个二维数组`dp`,其中`dp[i][j]`表示以`str1`的第`i`个字符和`str2`的第`j`个字符为结尾的最长公共子串的长度。当`str1.charAt(i-1) == str2.charAt(j-1)`时,表示`str1`和`str2`的第`i`和`j`个字符相同,因此可以通过`dp[i-1][j-1]`得到以`str1`的第`i-1`个字符和`str2`的第`j-1`个字符为结尾的最长公共子串长度,并加上当前相同的字符,即可得到以`str1`的第`i`个字符和`str2`的第`j`个字符为结尾的最长公共子串的长度。同时,需要记录每次更新的最大长度,并在遍历完成后返回该长度。
阅读全文
相关推荐
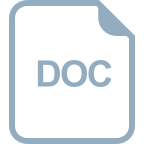
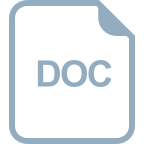
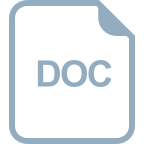









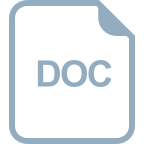
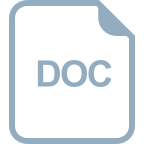
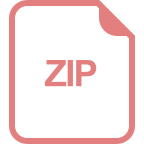
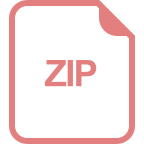