如何用torch搭建一个简单的神经网络模型
时间: 2024-05-30 10:13:32 浏览: 148
您好,使用torch搭建一个简单神经网络模型可以遵循以下步骤:
1. 导入所需的库
import torch
import torch.nn as nn
import torch.nn.functional as F
2. 创建一个自定义的神经网络模型类
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
self.fc1 = nn.Linear(5, 10) # 创建一个Linear层 输入5个特征,输出10个特征
self.fc2 = nn.Linear(10, 2) # 创建一个Linear层 输入10个特征,输出2个特征
def forward(self, x):
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
3. 创建一个训练数据集
X = torch.randn(100, 5) # 创建100个样本,每个样本包含5个特征
y = torch.randint(high=2, size=(100,)) # 随机生成y值
4. 创建一个模型实例,并定义优化器和损失函数
net = SimpleNet() # 创建一个模型实例
optimizer = torch.optim.SGD(net.parameters(), lr=0.01) # 使用随机梯度下降法作为优化算法
criterion = nn.CrossEntropyLoss() # 使用交叉熵作为损失函数
5. 训练模型
for epoch in range(1000):
optimizer.zero_grad() # 清除梯度
output = net(X) # 执行前向计算
loss = criterion(output, y) # 计算loss
loss.backward() # 计算梯度
optimizer.step() # 更新参数
6. 使用模型进行预测
with torch.no_grad():
new_x = torch.Tensor([[1, 2, 3, 4, 5], [5, 4, 3, 2, 1]]) # 创建两个测试样本
new_y = net(new_x) # 执行前向计算进行预测
print(new_y) # 输出预测结果
以上就是使用torch搭建一个简单的神经网络模型的步骤,希望能够对您有所帮助!
阅读全文
相关推荐
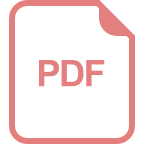
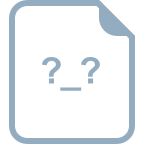


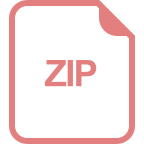
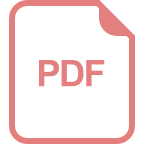
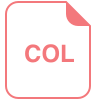
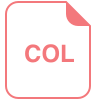
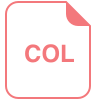








