用C++定义Point类描述点的坐标,可以输出坐标,可以求两个点之间的距离。 主函数声明Point类数组Point p[5],输入5个点的坐标,根据5个点与原点[0,0]之间的距离,由近至远排序输出距离值。 【输入形式】 5个点坐标 【输出形式】 排序后的距离值 【样例输入】 2 3 1 3 6 3 8 7 4 5
时间: 2024-02-09 21:11:46 浏览: 88
以下是C++的代码实现:
```c++
#include <iostream>
#include <cmath>
#include <algorithm>
using namespace std;
class Point {
public:
Point(double x = 0, double y = 0) : _x(x), _y(y) {}
double distance() const { return sqrt(_x * _x + _y * _y); }
void print() const { cout << "(" << _x << ", " << _y << ")"; }
bool operator < (const Point& other) const { return distance() < other.distance(); }
private:
double _x, _y;
};
int main() {
Point p[5];
for (int i = 0; i < 5; i++) {
double x, y;
cin >> x >> y;
p[i] = Point(x, y);
}
sort(p, p+5);
for (int i = 0; i < 5; i++) {
p[i].print();
cout << "距离原点的距离:" << p[i].distance() << endl;
}
return 0;
}
```
在这个程序中,我们声明了一个Point类,它有两个私有成员变量x和y,表示点的坐标。我们在类中定义了两个公有成员函数,distance()用于计算点到原点的距离,print()用于输出点的坐标。
在主函数中,我们声明了一个Point类型的数组p,并使用循环读入5个点的坐标。然后,我们使用sort函数将数组p中的元素按照距离由近至远排序。最后,我们再次用循环输出每个点的坐标和距离原点的距离。
注意,我们在Point类中重载了小于号运算符<,这样在sort函数中就可以直接使用了。
阅读全文
相关推荐
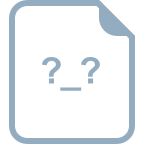















