写代码c++:定义Point类描述点的坐标,可以输出坐标,可以求两个点之间的距离。 主函数声明Point类数组Point p[5],输入5个点的坐标,根据5个点与原点[0,0]之间的距离,由近至远排序输出距离值。 【输入形式】 5个点坐标 【输出形式】 排序后的距离值 【样例输入】 2 3 1 3 6 3 8 7 4 5 【样例输出】 与原点的距离: 3.16 3.61 6.40 6.71 10.63
时间: 2024-02-18 07:59:02 浏览: 28
以下是C++代码实现:
```cpp
#include <iostream>
#include <cmath>
#include <algorithm>
using namespace std;
class Point {
public:
double x, y;
Point() {}
Point(double _x, double _y) : x(_x), y(_y) {}
void print() const {
cout << "(" << x << ", " << y << ")";
}
double distanceTo(const Point& p) const {
double dx = x - p.x, dy = y - p.y;
return sqrt(dx * dx + dy * dy);
}
};
bool cmp(const Point& p1, const Point& p2) {
double dist1 = p1.distanceTo(Point(0, 0));
double dist2 = p2.distanceTo(Point(0, 0));
return dist1 < dist2;
}
int main() {
Point p[5];
for (int i = 0; i < 5; ++i) {
cin >> p[i].x >> p[i].y;
}
sort(p, p + 5, cmp);
cout << "与原点的距离:\n";
for (int i = 0; i < 5; ++i) {
cout << p[i].distanceTo(Point(0, 0)) << " ";
}
cout << endl;
return 0;
}
```
代码思路:
定义一个Point类来描述点的坐标,包含x和y两个成员变量,以及输出坐标和求两点之间距离的函数。
在主函数中,定义一个Point类数组Point p[5],并且输入5个点的坐标。然后,利用sort函数对这5个点进行排序,排序的规则是按照与原点的距离由近至远排序。最后,输出排序后的距离值即可。
需要注意的是,这里我们自己定义了一个cmp函数来作为sort函数的比较函数。在cmp函数中,我们利用Point类的distanceTo函数来计算每个点与原点之间的距离,然后按照距离从小到大排序。
相关推荐
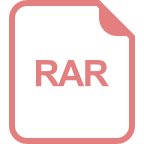
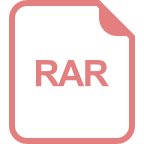














