C++题目58:对点坐标与原点距离排序 定义Point类描述点的坐标,可以输出坐标,可以求两个点之间的距离。 主函数声明Point类数组Point p[5],输入5个点的坐标,根据5个点与原点[0,0]之间的距离,由近至远排序输出距离值。所以vector
时间: 2024-02-11 17:05:46 浏览: 22
实现方式如下:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
#include <cmath>
using namespace std;
class Point {
public:
Point(double x, double y) : x(x), y(y) {}
double distanceToOrigin() const {
return sqrt(x * x + y * y);
}
void print() const {
cout << "(" << x << ", " << y << ")";
}
bool operator<(const Point& other) const {
return distanceToOrigin() < other.distanceToOrigin();
}
private:
double x, y;
};
int main() {
vector<Point> points;
for (int i = 0; i < 5; i++) {
double x, y;
cin >> x >> y;
points.push_back(Point(x, y));
}
sort(points.begin(), points.end());
for (int i = 0; i < 5; i++) {
points[i].print();
cout << " Distance to origin: " << points[i].distanceToOrigin() << endl;
}
return 0;
}
```
首先我们定义了一个 Point 类,用于描述点的坐标,包括坐标的输入输出和计算两点之间距离的方法。
在主函数中,我们使用 vector 存储 5 个点,并使用 sort 函数进行排序。由于我们在 Point 类中重载了小于运算符,因此 sort 函数会默认按照点到原点的距离进行排序。
最后,我们遍历排序后的点,输出它们的坐标和到原点的距离。
相关推荐
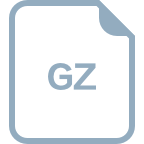
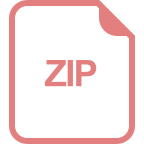














