c语言汉字编码转换
时间: 2023-07-02 10:11:19 浏览: 139
在 C 语言中,可以使用 wchar_t 类型来存储 Unicode 编码的字符。如果要将汉字编码转换为 Unicode 编码,可以使用一些库函数,如 mbstowcs() 或 MultiByteToWideChar()。
mbstowcs() 函数可以将多字节字符串转换为宽字符字符串,其语法如下:
```
size_t mbstowcs(wchar_t *dest, const char *src, size_t n);
```
其中,dest 表示转换后的宽字符字符串,src 表示待转换的多字节字符串,n 表示最多转换的字符数。
示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <wchar.h>
#include <locale.h>
int main() {
// 设置本地化环境
setlocale(LC_ALL, "");
// 待转换的汉字字符串
char *str = "你好,世界!";
// 分配存储宽字符字符串的空间
size_t len = strlen(str);
wchar_t *wstr = (wchar_t *)malloc((len + 1) * sizeof(wchar_t));
// 转换为宽字符字符串
size_t ret = mbstowcs(wstr, str, len + 1);
// 输出转换结果
wprintf(L"宽字符字符串:%ls\n", wstr);
// 释放内存
free(wstr);
return 0;
}
```
MultiByteToWideChar() 函数是 Windows 系统中的 API 函数,可以将 ANSI 编码或 UTF-8 编码转换为 Unicode 编码。其语法如下:
```
int MultiByteToWideChar(UINT CodePage, DWORD dwFlags, LPCSTR lpMultiByteStr, int cbMultiByte, LPWSTR lpWideCharStr, int cchWideChar);
```
其中,CodePage 表示待转换的字符集编码,dwFlags 表示转换选项,lpMultiByteStr 表示待转换的多字节字符串,cbMultiByte 表示待转换的多字节字符串长度(单位为字节),lpWideCharStr 表示转换后的宽字符字符串,cchWideChar 表示存储转换后的宽字符字符串的缓冲区大小(单位为字符)。
示例代码:
```c
#include <stdio.h>
#include <windows.h>
int main() {
// 待转换的汉字字符串
char *str = "你好,世界!";
// 分配存储宽字符字符串的空间
int len = strlen(str);
int wlen = MultiByteToWideChar(CP_UTF8, 0, str, len, NULL, 0);
wchar_t *wstr = (wchar_t *)malloc((wlen + 1) * sizeof(wchar_t));
// 转换为宽字符字符串
MultiByteToWideChar(CP_UTF8, 0, str, len, wstr, wlen + 1);
// 输出转换结果
wprintf(L"宽字符字符串:%ls\n", wstr);
// 释放内存
free(wstr);
return 0;
}
```
阅读全文
相关推荐
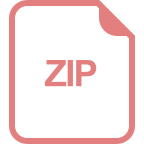
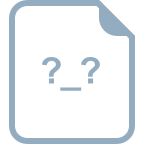

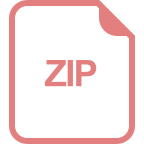
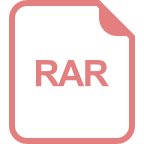
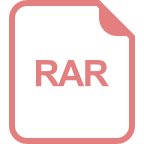
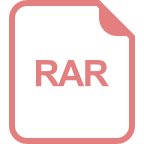
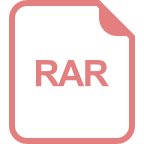
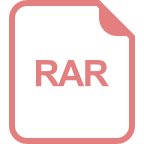
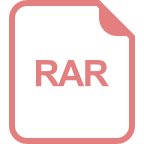
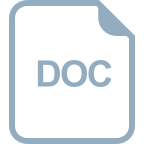
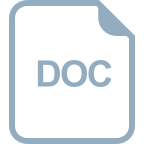
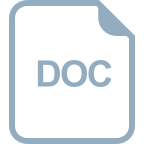
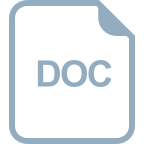


