用C实现remosaic
时间: 2024-03-20 11:41:10 浏览: 19
以下是使用 C 语言实现的 remosaic 函数示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 马赛克单元格大小
#define CELL_SIZE 2
void remosaic(unsigned char* image, int width, int height, const char* mosaic_pattern, unsigned char* remosaic_image) {
int row, col, channel, cell_row, cell_col;
int channels[4];
int channel_vals[4];
int offset[4][2] = {{0, 0}, {0, 1}, {1, 0}, {1, 1}};
int R_offset, G_offset, B_offset;
int mosaic_pattern_len = strlen(mosaic_pattern);
// 根据马赛克模式设置颜色通道顺序
if (strcmp(mosaic_pattern, "RGGB") == 0) {
channels[0] = 0;
channels[1] = 1;
channels[2] = 2;
channels[3] = 1;
R_offset = 0;
G_offset = 1;
B_offset = 2;
} else if (strcmp(mosaic_pattern, "GBRG") == 0) {
channels[0] = 1;
channels[1] = 0;
channels[2] = 1;
channels[3] = 2;
R_offset = 2;
G_offset = 1;
B_offset = 0;
} else if (strcmp(mosaic_pattern, "GRBG") == 0) {
channels[0] = 1;
channels[1] = 2;
channels[2] = 0;
channels[3] = 1;
R_offset = 1;
G_offset = 2;
B_offset = 0;
} else if (strcmp(mosaic_pattern, "BGGR") == 0) {
channels[0] = 2;
channels[1] = 1;
channels[2] = 1;
channels[3] = 0;
R_offset = 2;
G_offset = 0;
B_offset = 1;
} else {
printf("Invalid mosaic pattern!\n");
return;
}
// 逐个马赛克单元格处理
for (row = 0; row < height; row += CELL_SIZE) {
for (col = 0; col < width; col += CELL_SIZE) {
// 获取该单元格的颜色通道值
for (channel = 0; channel < 4; channel++) {
cell_row = row + offset[channel][0];
cell_col = col + offset[channel][1];
channel_vals[channel] = image[(cell_row * width + cell_col) * 3 + channels[channel]];
}
// 按照马赛克模式重新排列颜色通道值
for (channel = 0; channel < 4; channel++) {
channel_vals[channel] = channel_vals[channels[channel]];
}
// 将颜色通道值反映到输出图像对应的像素中
for (cell_row = row; cell_row < row + CELL_SIZE; cell_row++) {
for (cell_col = col; cell_col < col + CELL_SIZE; cell_col++) {
remosaic_image[(cell_row * width + cell_col) * 3 + R_offset] = channel_vals[0];
remosaic_image[(cell_row * width + cell_col) * 3 + G_offset] = channel_vals[1];
remosaic_image[(cell_row * width + cell_col) * 3 + B_offset] = channel_vals[2];
}
}
}
}
}
int main() {
// 读取原始图像
FILE* fp = fopen("image.raw", "rb");
int width = 640;
int height = 480;
unsigned char* image = (unsigned char*)malloc(width * height * 3);
fread(image, 1, width * height * 3, fp);
fclose(fp);
// 执行 remosaic 操作
char mosaic_pattern[] = "RGGB";
unsigned char* remosaic_image = (unsigned char*)malloc(width * height * 3);
remosaic(image, width, height, mosaic_pattern, remosaic_image);
// 写入输出图像
fp = fopen("remosaic.raw", "wb");
fwrite(remosaic_image, 1, width * height * 3, fp);
fclose(fp);
free(image);
free(remosaic_image);
return 0;
}
```
该示例使用了一个 `remosaic` 函数来执行 remosaic 操作,其输入参数包括原始图像、图像宽度、图像高度、马赛克模式和输出图像。函数的实现方式与 Python 版本类似,也是逐个马赛克单元格处理,获取其颜色通道值并按照马赛克模式重新排列颜色通道值,最后将颜色通道值反映到输出图像对应的像素中。注意,在 C 语言中,需要通过指针来传递数组参数。
相关推荐
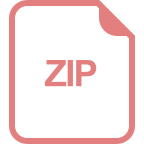












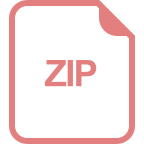
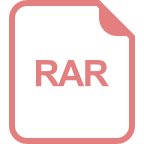
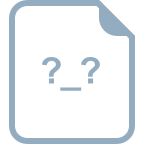