OpenGL Cohen-Sutherland code
时间: 2024-01-16 15:05:16 浏览: 27
Here is an example code for the Cohen-Sutherland line clipping algorithm using OpenGL:
```
#include <GL/glut.h>
// Define the clipping region constants
const int INSIDE = 0;
const int LEFT = 1;
const int RIGHT = 2;
const int BOTTOM = 4;
const int TOP = 8;
// Define the window coordinates
const int XMIN = 50;
const int XMAX = 250;
const int YMIN = 50;
const int YMAX = 250;
// Define the line coordinates
int x1 = 30;
int y1 = 100;
int x2 = 200;
int y2 = 300;
// Compute the outcode for a point
int computeOutCode(int x, int y) {
int code = INSIDE;
if (x < XMIN) {
code |= LEFT;
} else if (x > XMAX) {
code |= RIGHT;
}
if (y < YMIN) {
code |= BOTTOM;
} else if (y > YMAX) {
code |= TOP;
}
return code;
}
// Clip the line using the Cohen-Sutherland algorithm
void cohenSutherlandLineClip() {
int outcode1 = computeOutCode(x1, y1);
int outcode2 = computeOutCode(x2, y2);
bool accept = false;
while (true) {
if (!(outcode1 | outcode2)) {
accept = true;
break;
} else if (outcode1 & outcode2) {
break;
} else {
int x, y;
int outcodeOut = outcode1 ? outcode1 : outcode2;
if (outcodeOut & TOP) {
x = x1 + (x2 - x1) * (YMAX - y1) / (y2 - y1);
y = YMAX;
} else if (outcodeOut & BOTTOM) {
x = x1 + (x2 - x1) * (YMIN - y1) / (y2 - y1);
y = YMIN;
} else if (outcodeOut & RIGHT) {
y = y1 + (y2 - y1) * (XMAX - x1) / (x2 - x1);
x = XMAX;
} else {
y = y1 + (y2 - y1) * (XMIN - x1) / (x2 - x1);
x = XMIN;
}
if (outcodeOut == outcode1) {
x1 = x;
y1 = y;
outcode1 = computeOutCode(x1, y1);
} else {
x2 = x;
y2 = y;
outcode2 = computeOutCode(x2, y2);
}
}
}
if (accept) {
glColor3f(0.0f, 1.0f, 0.0f); // Green color for the clipped line
} else {
glColor3f(1.0f, 0.0f, 0.0f); // Red color for the rejected line
}
glBegin(GL_LINES);
glVertex2i(x1, y1);
glVertex2i(x2, y2);
glEnd();
}
// Display function
void display() {
glClear(GL_COLOR_BUFFER_BIT);
// Draw the window boundaries
glColor3f(1.0f, 1.0f, 1.0f); // White color for the window
glBegin(GL_LINE_LOOP);
glVertex2i(XMIN, YMIN);
glVertex2i(XMAX, YMIN);
glVertex2i(XMAX, YMAX);
glVertex2i(XMIN, YMAX);
glEnd();
// Draw the original line
glColor3f(1.0f, 0.0f, 0.0f); // Red color for the original line
glBegin(GL_LINES);
glVertex2i(x1, y1);
glVertex2i(x2, y2);
glEnd();
// Clip the line using the Cohen-Sutherland algorithm
cohenSutherlandLineClip();
glFlush();
}
// Main function
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowSize(300, 300);
glutInitWindowPosition(100, 100);
glutCreateWindow("Cohen-Sutherland Line Clipping Algorithm");
glClearColor(0.0f, 0.0f, 0.0f, 0.0f);
gluOrtho2D(0, 300, 0, 300);
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
```
相关推荐
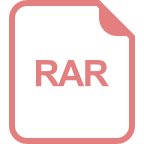














