windows下c++使用ghostscript的api接口静默打印pdf
时间: 2024-03-13 16:46:26 浏览: 230
使用Ghostscript的API接口可以在Windows下实现静默打印PDF文件。Ghostscript是一个开源的PDF处理引擎,它提供了C/C++接口,可以在程序中调用相应的函数实现PDF打印功能。
下面是一个简单的示例代码,演示如何使用Ghostscript的API接口实现静默打印PDF文件:
```c++
#include <stdio.h>
#include <windows.h>
#include "gdiplus.h"
#include "gs/gsapi.h"
#pragma comment(lib, "gdiplus.lib")
#pragma comment(lib, "gsdll32.lib")
void print_pdf(const wchar_t* filename, const wchar_t* printer_name)
{
// 初始化Ghostscript API
int argc = 3;
const char* argv[3] = {
"gsdll32.dll",
"-dNOPAUSE",
"-dBATCH"
};
GSAPI_HANDLE gs_handle;
gsapi_new_instance(&gs_handle, NULL);
// 设置输出打印机
char printer[256] = { 0 };
char port[256] = { 0 };
WideCharToMultiByte(CP_ACP, 0, printer_name, -1, printer, sizeof(printer), NULL, NULL);
PRINTER_INFO_2* pinfo = NULL;
DWORD num_printers = 0;
EnumPrinters(PRINTER_ENUM_LOCAL, NULL, 2, NULL, 0, &num_printers, NULL);
pinfo = (PRINTER_INFO_2*)malloc(num_printers * sizeof(PRINTER_INFO_2));
EnumPrinters(PRINTER_ENUM_LOCAL, NULL, 2, (LPBYTE)pinfo, num_printers * sizeof(PRINTER_INFO_2), &num_printers, NULL);
for (DWORD i = 0; i < num_printers; ++i) {
if (strcmp(pinfo[i].pPrinterName, printer) == 0) {
sprintf_s(port, sizeof(port), "%s", pinfo[i].pPortName);
break;
}
}
free(pinfo);
gsapi_set_arg_encoding(gs_handle, GS_ARG_ENCODING_UTF8);
gsapi_set_stdio(gs_handle, NULL, NULL, NULL);
gsapi_init_with_args(gs_handle, argc, (char**)argv);
gsapi_set_arg_encoding(gs_handle, GS_ARG_ENCODING_UTF8);
gsapi_set_stdio(gs_handle, NULL, NULL, NULL);
gsapi_set_poll(gs_handle, NULL);
gsapi_set_display_callback(gs_handle, NULL);
gsapi_set_stdin(gs_handle, NULL);
gsapi_set_stdout(gs_handle, NULL);
// 打印PDF文件
wchar_t command[1024] = { 0 };
swprintf_s(command, sizeof(command), L"-dPrinted -dBATCH -dNOPAUSE -dNOSAFER -q -dNumCopies=1 -sDEVICE=mswinpr2 -sOutputFile=\"%%printer%s\" \"%s\"", port, filename);
char* argv2[64] = { 0 };
int argc2 = 0;
const wchar_t* p = command;
while (*p) {
argv2[argc2++] = (char*)p;
while (*p && !iswspace(*p))
p++;
if (*p)
*p++ = 0;
}
gsapi_exit(gs_handle);
gsapi_delete_instance(gs_handle);
}
int main()
{
Gdiplus::GdiplusStartupInput gdiplusStartupInput;
ULONG_PTR gdiplusToken;
Gdiplus::GdiplusStartup(&gdiplusToken, &gdiplusStartupInput, NULL);
wchar_t filename[] = L"C:\\test.pdf";
wchar_t printer_name[] = L"Microsoft Print to PDF";
print_pdf(filename, printer_name);
Gdiplus::GdiplusShutdown(gdiplusToken);
return 0;
}
```
需要注意的是,使用Ghostscript打印PDF文件需要确保Ghostscript已经正确安装并设置好环境变量。另外,在打印PDF文件前,需要先通过Ghostscript API初始化Ghostscript引擎,并设置输出打印机等参数。最后,调用Ghostscript API打印PDF文件即可。
阅读全文
相关推荐





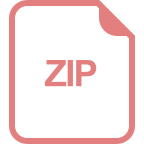
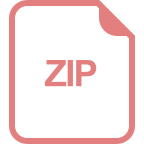
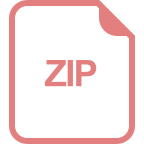
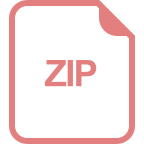
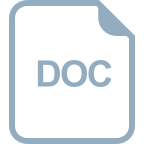
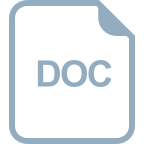



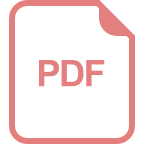
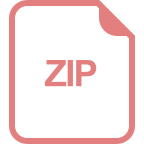