c++在windows平台下使用ghostscript的api接口静默打印pdf
时间: 2024-06-11 07:08:51 浏览: 294
Ghostscript是一款开源的PostScript和PDF解释器,可以在Windows平台下使用其API接口进行静默打印PDF。
以下是实现步骤:
1. 下载并安装Ghostscript
从Ghostscript官网(https://www.ghostscript.com/download/gsdnld.html)下载Windows平台下的安装程序,安装到本地计算机上。
2. 编写代码
使用Ghostscript提供的API接口,在代码中实现PDF文件的静默打印。以下是一个示例代码:
```
#include <stdio.h>
#include "gsapi.h"
int main(int argc, char *argv[])
{
const char *pdfFile = "C:\\test.pdf";
const char *printerName = "My Printer";
const char *deviceName = "mswinpr2";
const char *outputFile = "C:\\test.prn";
const char *outputFormat = "PRN";
const char *args[] = {
"pdf2ps", "-sDEVICE=mswinpr2",
"-sOutputFile=C:\\test.prn",
"-dNOPAUSE", "-dBATCH", "-dSAFER",
"C:\\test.pdf", NULL
};
int argcCount = sizeof(args) / sizeof(args[0]) - 1;
gsapi_restart_ijs(NULL);
gsapi_revision_t revision;
gsapi_revision(&revision, sizeof(revision));
printf("Ghostscript version: %d.%d.%d\n", revision.product, revision.major, revision.minor);
gsapi_instance *instance;
int errorCode = gsapi_new_instance(&instance, NULL);
if (errorCode < 0) {
printf("Failed to create Ghostscript instance: %d\n", errorCode);
return -1;
}
gsapi_set_stdio(instance, stdin, stdout, stderr);
gsapi_set_poll(instance, NULL);
errorCode = gsapi_init_with_args(instance, argcCount, (char **)args);
if (errorCode < 0) {
printf("Failed to initialize Ghostscript instance: %d\n", errorCode);
return -1;
}
errorCode = gsapi_exit(instance);
if (errorCode < 0) {
printf("Failed to exit Ghostscript instance: %d\n", errorCode);
return -1;
}
// Send the output file to the printer
HANDLE printerHandle;
DOC_INFO_1 docInfo;
DWORD bytesWritten;
BOOL success;
success = OpenPrinter((LPSTR) printerName, &printerHandle, NULL);
if (!success) {
printf("Failed to open printer: %d\n", GetLastError());
return -1;
}
docInfo.pDocName = "Test Document";
docInfo.pOutputFile = NULL;
docInfo.pDatatype = "RAW";
success = StartDocPrinter(printerHandle, 1, (LPBYTE) &docInfo);
if (!success) {
printf("Failed to start print job: %d\n", GetLastError());
ClosePrinter(printerHandle);
return -1;
}
success = StartPagePrinter(printerHandle);
if (!success) {
printf("Failed to start page: %d\n", GetLastError());
EndDocPrinter(printerHandle);
ClosePrinter(printerHandle);
return -1;
}
HANDLE fileHandle = CreateFile(outputFile, GENERIC_READ, FILE_SHARE_READ, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (fileHandle == INVALID_HANDLE_VALUE) {
printf("Failed to open output file: %d\n", GetLastError());
EndPagePrinter(printerHandle);
EndDocPrinter(printerHandle);
ClosePrinter(printerHandle);
return -1;
}
BYTE buffer[4096];
DWORD bytesRead;
while (ReadFile(fileHandle, buffer, sizeof(buffer), &bytesRead, NULL)) {
if (bytesRead == 0) {
break;
}
success = WritePrinter(printerHandle, buffer, bytesRead, &bytesWritten);
if (!success) {
printf("Failed to write to printer: %d\n", GetLastError());
CloseHandle(fileHandle);
EndPagePrinter(printerHandle);
EndDocPrinter(printerHandle);
ClosePrinter(printerHandle);
return -1;
}
}
CloseHandle(fileHandle);
success = EndPagePrinter(printerHandle);
if (!success) {
printf("Failed to end page: %d\n", GetLastError());
EndDocPrinter(printerHandle);
ClosePrinter(printerHandle);
return -1;
}
success = EndDocPrinter(printerHandle);
if (!success) {
printf("Failed to end print job: %d\n", GetLastError());
ClosePrinter(printerHandle);
return -1;
}
ClosePrinter(printerHandle);
return 0;
}
```
3. 编译和运行
使用Visual Studio等编译器编译代码,生成可执行文件。在命令行中运行可执行文件,即可实现PDF文件的静默打印。
```
> ghostprint.exe
```
该示例代码将PDF文件转换为PRN格式的文件,并发送到指定的打印机进行打印。可以根据实际需求修改代码中的参数和变量。
阅读全文
相关推荐
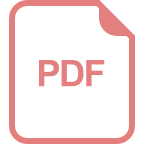

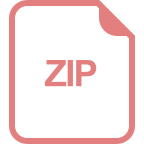
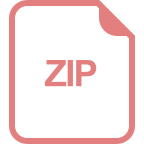
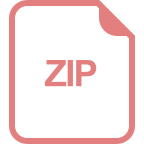
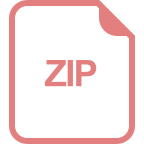
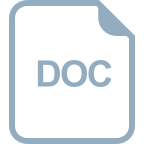
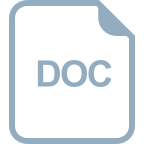






