Optix 光线追踪简单示例
时间: 2023-10-02 12:09:48 浏览: 58
好的,以下是一个简单的Optix光线追踪示例:
```C++
#include <optix.h>
#include <optixu/optixu_math_namespace.h>
#include <iostream>
using namespace optix;
int main()
{
// 初始化 Optix 上下文
RTcontext context = rtContextCreate();
// 设置 Optix 上下文的属性
RTvariable var;
RTprogram ray_gen_program;
RTprogram exception_program;
RTprogram miss_program;
// 创建 Optix 程序
const char* ptx = "..."; // 这里应该是 Optix 程序的 PTX 代码
ray_gen_program = rtProgramCreateFromPTXString(context, ptx, "ray_gen_function");
exception_program = rtProgramCreateFromPTXString(context, ptx, "exception_function");
miss_program = rtProgramCreateFromPTXString(context, ptx, "miss_function");
// 设置 Optix 程序的参数
RTsize width = 640;
RTsize height = 480;
rtContextSetAttribute(context, RT_CONTEXT_ATTRIBUTE_GPU_PAGING_FILE_SIZE, 2097152);
rtContextSetRayTypeCount(context, 1);
rtContextDeclareVariable(context, "width", &var);
rtContextDeclareVariable(context, "height", &var);
rtContextDeclareVariable(context, "output_buffer", &var);
rtVariableSet1ui(var, width);
rtVariableSet1ui(var, height);
rtVariableSetBuffer(var, output_buffer);
rtContextSetEntryPointCount(context, 1);
rtContextSetRayGenerationProgram(context, 0, ray_gen_program);
rtContextSetExceptionProgram(context, 0, exception_program);
rtContextSetMissProgram(context, 0, miss_program);
// 创建 Optix 输出缓冲区
RTbuffer output_buffer;
rtBufferCreate(context, RT_BUFFER_OUTPUT, &output_buffer);
rtBufferSetFormat(output_buffer, RT_FORMAT_FLOAT4);
rtBufferSetSize2D(output_buffer, width, height);
// 运行 Optix 上下文
rtContextValidate(context);
rtContextLaunch2D(context, 0, width, height);
// 读取 Optix 输出缓冲区
float4* data = reinterpret_cast<float4*>(rtBufferMap(output_buffer, RT_BUFFER_MAP_READ));
for (int j = 0; j < height; ++j)
{
for (int i = 0; i < width; ++i)
{
std::cout << "Pixel (" << i << ", " << j << "): (" << data[j * width + i].x << ", " << data[j * width + i].y << ", " << data[j * width + i].z << ")" << std::endl;
}
}
rtBufferUnmap(output_buffer);
// 清理资源
rtBufferDestroy(output_buffer);
rtProgramDestroy(ray_gen_program);
rtProgramDestroy(exception_program);
rtProgramDestroy(miss_program);
rtContextDestroy(context);
return 0;
}
```
这个示例中,我们首先创建了一个 Optix 上下文 `context`,然后创建了三个 Optix 程序 `ray_gen_program`、`exception_program`、`miss_program`,并设置了它们的属性。接着,我们创建了一个输出缓冲区 `output_buffer`,并设置了 Optix 上下文的属性,最后运行 Optix 上下文,读取输出缓冲区中的数据,清理资源。
相关推荐
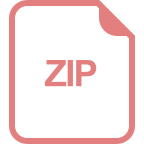
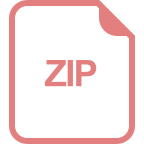














