yolov7模型调用摄像头
时间: 2023-09-26 13:05:49 浏览: 100
要使用YOLOv7模型调用摄像头,您需要使用OpenCV库来捕获视频流并将其传递给YOLOv7模型进行对象检测。以下是一个简单的示例代码:
```python
import cv2
import torch
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords, plot_one_box
from utils.datasets import letterbox
# 加载YOLOv7模型
weights = 'path/to/weights.pt'
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = attempt_load(weights, map_location=device)
# 设置输入分辨率和阈值等参数
input_size = 640
conf_threshold = 0.5
iou_threshold = 0.45
# 打开摄像头并捕获视频流
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
# 将帧缩放到模型的输入大小
img = letterbox(frame, new_shape=input_size)[0]
img = img[:, :, ::-1].transpose(2, 0, 1)
img = np.ascontiguousarray(img)
img = torch.from_numpy(img).to(device)
img = img.float() / 255.0
# 运行模型进行对象检测
with torch.no_grad():
outputs = model(img.unsqueeze(0))
outputs = non_max_suppression(outputs, conf_threshold, iou_threshold)
# 绘制检测结果
for output in outputs:
if output is not None:
output[:, :4] = scale_coords(img.shape[2:], output[:, :4], frame.shape).round()
for x1, y1, x2, y2, conf, cls in output:
label = f'{model.names[int(cls)]} {conf:.2f}'
plot_one_box((x1, y1, x2, y2), frame, label=label)
# 显示视频流
cv2.imshow('Object Detection', frame)
if cv2.waitKey(1) == 27:
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
```
在这个示例中,我们使用了 `models.experimental.attempt_load` 函数来加载YOLOv7模型,然后使用 `utils.general.non_max_suppression` 函数对模型输出的边界框进行非最大抑制,再使用 `utils.datasets.letterbox` 函数将帧缩放到模型的输入大小。最后,我们使用OpenCV库来捕获视频流并将检测结果绘制在每一帧上,然后显示视频流。
相关推荐
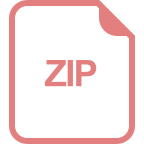
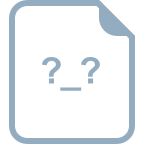














