yolov5调用电脑摄像头
时间: 2023-10-16 14:09:44 浏览: 64
使用Python和OpenCV库可以轻松地调用电脑摄像头并将视频流传递到YOLOv5模型中进行目标检测。
以下是一个简单的示例代码:
```python
import cv2
import torch
from torch.utils.data import DataLoader
from models.experimental import attempt_load
from utils.datasets import LoadStreams
from utils.general import check_img_size, check_requirements, non_max_suppression, scale_coords, set_logging
from utils.plots import plot_one_box
from utils.torch_utils import select_device, time_sync
# 加载YOLOv5模型
weights = 'yolov5s.pt'
device = select_device('')
model = attempt_load(weights, map_location=device)
stride = int(model.stride.max())
# 设置日志和图像大小
set_logging()
imgsz = check_img_size(640, s=stride)
# 加载摄像头
cap = cv2.VideoCapture(0)
# 循环读取视频流并进行目标检测
while cap.isOpened():
# 读取一帧图像
ret, frame = cap.read()
if not ret:
break
img = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 图像预处理
img = cv2.resize(img, imgsz)
img = torch.from_numpy(img).to(device)
img = img.float() / 255.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
# YOLOv5模型推理
t1 = time_sync()
pred = model(img, augment=False)[0]
pred = non_max_suppression(pred, 0.4, 0.5, agnostic=False, max_det=1000)
t2 = time_sync()
# 绘制检测结果
for det in pred:
if det is not None and len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], frame.shape).round()
for *xyxy, conf, cls in reversed(det):
label = f'{model.names[int(cls)]} {conf:.2f}'
plot_one_box(xyxy, frame, label=label, color=(0, 255, 0), line_thickness=3)
# 显示图像
cv2.imshow('YOLOv5', frame)
# 按'q'键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
此示例代码将打开电脑摄像头并显示实时视频流。YOLOv5模型将在每帧图像上运行目标检测,并将结果绘制在图像上。按下“q”键将退出程序。
相关推荐










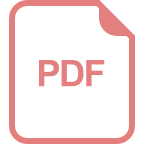