一个yolov5模型如何调用摄像头
时间: 2023-12-25 07:48:19 浏览: 105
要调用摄像头,可以使用OpenCV库来捕捉视频流,并将其传递给Yolov5模型进行对象检测。
以下是一个使用OpenCV和Yolov5模型的Python代码示例,可以从摄像头捕获视频流并进行对象检测:
```
import cv2
import torch
import numpy as np
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.datasets import letterbox
# Load Yolov5 model
model = attempt_load('yolov5s.pt', map_location=torch.device('cpu'))
# Set device
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
# Set input size
img_size = 640
# Set confidence threshold
conf_thresh = 0.5
# Set NMS threshold
nms_thresh = 0.4
# Set classes
classes = ['person', 'car', 'truck', 'bus', 'motorcycle']
# Initialize video capture
cap = cv2.VideoCapture(0)
while True:
# Read frame from camera
ret, frame = cap.read()
# If frame is not readable, break loop
if not ret:
break
# Convert frame to RGB
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# Resize and pad frame to input size
img, ratio, pad = letterbox(frame, new_shape=img_size)
# Convert image to tensor
img = torch.from_numpy(img.transpose(2, 0, 1)).unsqueeze(0).float()
# Normalize image
img /= 255.0
# Send image to device
img = img.to(device)
# Apply Yolov5 model to image
pred = model(img)[0]
# Apply non-maximum suppression
pred = non_max_suppression(pred, conf_thresh, nms_thresh)
# Process detections
for det in pred:
# If detections exist
if det is not None and len(det):
# Rescale detections to original frame size
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], frame.shape).round()
# Iterate over detections
for *xyxy, conf, cls in reversed(det):
# Get class name
cls = classes[int(cls)]
# Draw bounding box
cv2.rectangle(frame, tuple(xyxy[:2]), tuple(xyxy[2:]), (0, 255, 0), 2)
# Draw class label
cv2.putText(frame, cls, (xyxy[0], xyxy[1] - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.6, (0, 255, 0), 2)
# Display frame
cv2.imshow('Frame', frame)
# Exit on key press
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release video capture and destroy all windows
cap.release()
cv2.destroyAllWindows()
```
在上面的示例中,我们首先加载Yolov5模型,然后设置设备(在这里我们使用CPU)。然后,我们定义输入图像的大小、置信度阈值、NMS阈值和类别列表。接下来,我们初始化视频捕获器并进入循环。在每次循环中,我们从摄像头读取一帧,并将其转换为RGB格式。然后,我们将帧调整为指定的输入大小,并将其转换为PyTorch张量。我们还将图像归一化,并将其发送到设备。接下来,我们将Yolov5模型应用到图像上,并应用非极大值抑制。最后,我们对所有检测进行迭代,并在原始帧上绘制边界框和类别标签。最后,我们显示帧,并在按下“q”键时退出循环。
阅读全文
相关推荐
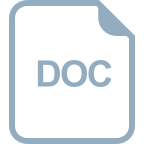
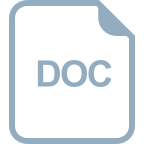
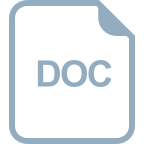


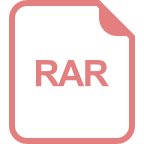












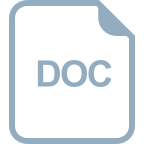