完成以下程序:进程A向消息队列发送消息“hello,world” 进程B从消息队列读取消息,并打印。 进程C向消息队列发送“自己在姓名” 进程D从消息队列中取出姓名字符串,并打印
时间: 2024-05-12 19:12:52 浏览: 177
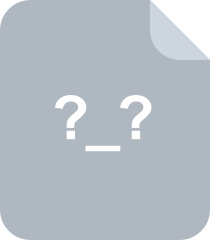
消息队列程序,进程间通信。
下面是基于Linux系统下的C语言代码实现:
进程A发送消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
} msg_buf;
int main() {
int msgid;
msg_buf msg;
// 创建消息队列
msgid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 发送消息
msg.mtype = 1;
strcpy(msg.mtext, "hello, world");
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd error");
exit(EXIT_FAILURE);
}
return 0;
}
```
进程B接收消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
} msg_buf;
int main() {
int msgid;
msg_buf msg;
// 获取消息队列
msgid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 接收消息
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 1, 0) == -1) {
perror("msgrcv error");
exit(EXIT_FAILURE);
}
printf("%s\n", msg.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl error");
exit(EXIT_FAILURE);
}
return 0;
}
```
进程C发送姓名消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
} msg_buf;
int main() {
int msgid;
msg_buf msg;
// 创建消息队列
msgid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 发送消息
msg.mtype = 1;
strcpy(msg.mtext, "Jack");
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd error");
exit(EXIT_FAILURE);
}
return 0;
}
```
进程D接收姓名消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
} msg_buf;
int main() {
int msgid;
msg_buf msg;
// 获取消息队列
msgid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 接收消息
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 1, 0) == -1) {
perror("msgrcv error");
exit(EXIT_FAILURE);
}
printf("%s\n", msg.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl error");
exit(EXIT_FAILURE);
}
return 0;
}
```
以上代码实现了进程间通过消息队列进行通信的功能,具体的原理和实现细节请参考Linux系统下的相关文档。
阅读全文
相关推荐
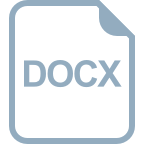
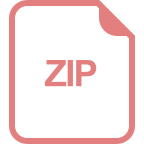

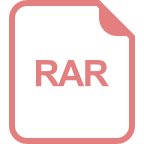
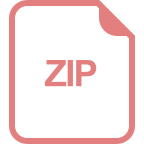
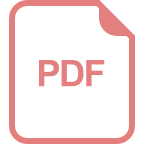
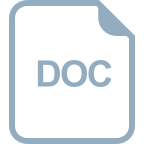
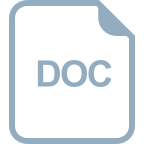
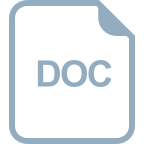
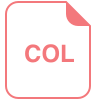
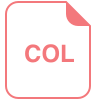
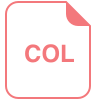
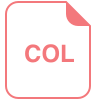
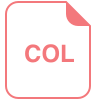
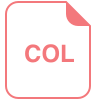


