from tkinter import * import time class ChatInterface(Frame): def __init__(self, master=None): Frame.__init__(self, master) self.master = master self.initialise() def initialise(self): self.grid() self.entry = Entry(self) self.entry.bind("<Return>", self.send) self.entry.grid(row=0, column=0, sticky=NSEW) self.submit_button = Button(self, text="Send", command=self.send) self.submit_button.grid(row=0, column=1) self.message_box = Text(self, state=DISABLED) self.message_box.grid(row=1, column=0, columnspan=2, sticky=NSEW) class ChatBot: def __init__(self): self.chat_history = [] self.current_question = "" self.response = "" def get_response(self, user_input): # Your code for generating a response goes here return response def send(self, event=None): message = self.entry.get() chat_bot.chat_history.append("You: " + message) self.message_box.configure(state=NORMAL) self.message_box.insert(END, "You: " + message + "\n") self.entry.delete(0, END) self.message_box.configure(state=DISABLED) time.sleep(0.5) self.message_box.configure(state=NORMAL) chat_bot_response = chat_bot.get_response(message) self.message_box.insert(END, "ChatBot: " + chat_bot_response + "\n") chat_bot.chat_history.append("ChatBot: " + chat_bot_response) self.message_box.configure(state=DISABLED) root = Tk() root.title("ChatBot") chat_bot = ChatBot() ChatInterface(root) root.mainloop()这个代码是否有bug
时间: 2023-12-05 08:04:25 浏览: 122
是的,这个代码有一些bug:
1. ChatBot类没有被实例化,需要在ChatInterface类中实例化ChatBot类。
2. get_response方法中的response变量没有被定义,应该将其替换为self.response。
3. send方法中的time.sleep(0.5)会阻塞GUI线程,应该避免使用。
4. ChatInterface类的initialise方法中没有设置self.message_box的高度和宽度,需要指定。
下面是修改后的代码:
```python
from tkinter import *
import time
class ChatInterface(Frame):
def __init__(self, master=None):
Frame.__init__(self, master)
self.master = master
self.chat_bot = ChatBot()
self.initialise()
def initialise(self):
self.grid()
self.entry = Entry(self)
self.entry.bind("<Return>", self.send)
self.entry.grid(row=0, column=0, sticky=NSEW)
self.submit_button = Button(self, text="Send", command=self.send)
self.submit_button.grid(row=0, column=1)
self.message_box = Text(self, state=DISABLED, height=20, width=50)
self.message_box.grid(row=1, column=0, columnspan=2, sticky=NSEW)
def send(self, event=None):
message = self.entry.get()
self.chat_bot.chat_history.append("You: " + message)
self.message_box.configure(state=NORMAL)
self.message_box.insert(END, "You: " + message + "\n")
self.entry.delete(0, END)
self.message_box.configure(state=DISABLED)
chat_bot_response = self.chat_bot.get_response(message)
self.message_box.configure(state=NORMAL)
self.message_box.insert(END, "ChatBot: " + chat_bot_response + "\n")
self.chat_bot.chat_history.append("ChatBot: " + chat_bot_response)
self.message_box.configure(state=DISABLED)
class ChatBot:
def __init__(self):
self.chat_history = []
self.current_question = ""
self.response = ""
def get_response(self, user_input):
# Your code for generating a response goes here
return self.response
root = Tk()
root.title("ChatBot")
ChatInterface(root)
root.mainloop()
```
阅读全文
相关推荐
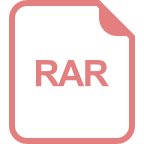
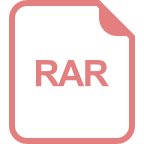
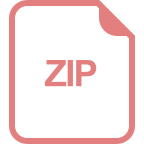















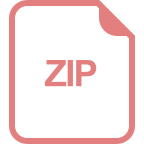