用bpso对红外光谱数据进行波段筛选的代码
时间: 2023-08-22 17:07:10 浏览: 67
以下是使用 BPSO(Binary Particle Swarm Optimization)算法进行红外光谱数据波段筛选的示例代码:
```python
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
from pyswarm import pso
# 读取红外光谱数据
data = pd.read_csv('infrared_data.csv')
# 将数据分为训练集和测试集
train_data, test_data, train_label, test_label = train_test_split(
data.iloc[:,:-1], data.iloc[:,-1], test_size=0.3, random_state=42)
# 定义适应度函数
def fitness_function(position, train_data, test_data, train_label, test_label):
# 将位置向量转换为二进制矩阵,筛选出选择的波段
selected_bands = np.where(position == 1)[0]
selected_train_data = train_data.iloc[:,selected_bands]
selected_test_data = test_data.iloc[:,selected_bands]
# 训练随机森林模型并预测测试集结果
rf = RandomForestClassifier(n_estimators=100, random_state=42)
rf.fit(selected_train_data, train_label)
pred_label = rf.predict(selected_test_data)
# 返回精度作为适应度值
return -accuracy_score(test_label, pred_label)
# 定义变量的上下限和粒子群大小
lb = np.zeros(data.shape[1]-1)
ub = np.ones(data.shape[1]-1)
n_particles = 20
# 使用 BPSO 算法进行波段筛选
best_position, best_fitness = pso(fitness_function, lb, ub,
args=(train_data, test_data, train_label, test_label),
swarmsize=n_particles, maxiter=50)
# 输出最佳精度和选择的波段
print('Best Accuracy:', -best_fitness)
selected_bands = np.where(best_position == 1)[0]
print('Selected Bands:', selected_bands)
```
代码中使用了 `pyswarm` 库实现了 BPSO 算法,适应度函数使用了随机森林分类器对波段进行筛选和分类。最终输出了最佳精度和选择的波段。注意,这里为了方便起见,使用了负的精度作为适应度值,因为 BPSO 算法是寻找最小值。
相关推荐
















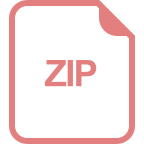
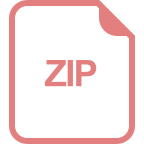